C++ Exercises: Print the first N numbers for a specific base
68. First N Numbers for a Specific Base
Write a program that will print the first N numbers for a specific base.
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int trm, bs, r, q, i, num; // Declare integer variables for terms, base, remainders, quotient, iteration, and number
// Display information about converting numbers to different bases
cout << "\n\n Print the first N numbers for a specific base:\n";
cout << " The number 11 in base 10 = 1*(10^1)+1*(10^0)=11" << endl;
cout << " Similarly the number 11 in base 7 = 1*(7^1)+1*(7^0)=8" << endl;
cout << "----------------------------------------------------------------\n";
cout << " Input the number of term: ";
cin >> trm; // Input the number of terms to display
cout << " Input the base: ";
cin >> bs; // Input the base for conversion
cout << " The numbers in base " << bs << " are: " << endl;
// Loop to calculate and display the first 'trm' numbers in base 'bs'
for (i = 1; i <= trm; i++)
{
r = i % bs; // Calculate remainder when 'i' is divided by 'bs' (base)
q = i / bs; // Calculate quotient when 'i' is divided by 'bs' (base)
num = q * 10 + r; // Convert the number 'i' to the specified base and store it in 'num'
cout << num << " "; // Display the number in the specified base
}
cout << endl;
}
Sample Output:
Print the first N numbers for a specific base: The number 11 in base 10 = 1*(10^1)+1*(10^0)=11 Similarly the number 11 in base 7 = 1*(7^1)+1*(7^0)=8 ---------------------------------------------------------------- Input the number of term: 15 Input the base: 9 The numbers in base 9 are: 1 2 3 4 5 6 7 8 10 11 12 13 14 15 16
Flowchart:
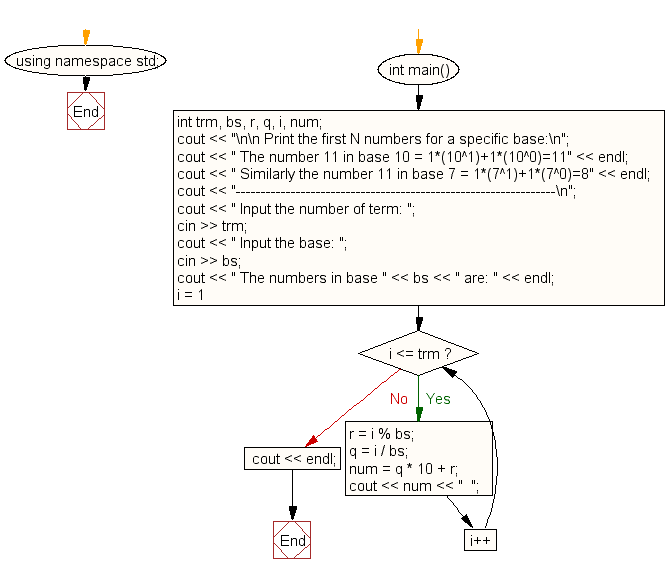
For more Practice: Solve these Related Problems:
- Write a C++ program to print the first N natural numbers in a given base by converting each number from decimal.
- Write a C++ program that reads N and a base value, then outputs the representation of numbers 1 through N in that base.
- Write a C++ program to generate a sequence of numbers in a specified base and display them in order.
- Write a C++ program that converts the first N numbers into a target base and prints the result as a space-separated list.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to calculate the sum of the series 1·2+2·3+3·4+4.5+5.6+.......
Next: Write a program in C++ to produce a square matrix with 0's down the main diagonal, 1's in the
entries just above and below the main diagonal, 2's above and below that, etc.
What is the difficulty level of this exercise?