C++ Exercises: Create a checkerboard pattern with the words "black" and "white"
C++ For Loop: Exercise-66 with Solution
Write code to create a checkerboard pattern with the words "black" and "white".
Visual Presentation:
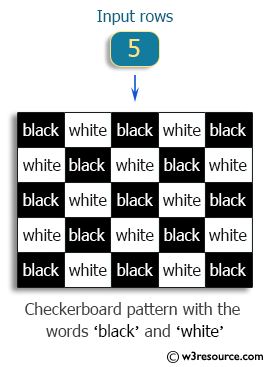
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, rows; // Declare variables for iteration and rows
string b, w, t; // Declare string variables b, w, and t for black, white, and temporary value storage
b = "black"; // Assign the string "black" to variable b
w = "white"; // Assign the string "white" to variable w
cout << "\n\n Display checkerboard pattern with the words 'black' and 'white':\n"; // Display description
cout << "---------------------------------------------------------------------\n";
cout << " Input number of rows: "; // Prompt user to input number of rows
cin >> rows; // Read the input number of rows from the user
// Loop to create a checkerboard pattern of words 'black' and 'white'
for (i = 1; i <= rows; i++)
{
// Loop to generate each row of the checkerboard pattern
for (j = 1; j <= rows; j++)
{
// Condition to alternate between 'black' and 'white' based on column position
if (j % 2 != 0)
{
cout << b; // Display 'black'
if (j < rows)
{
cout << "-"; // Display '-' to separate words
}
}
else if (j % 2 == 0)
{
cout << w; // Display 'white'
if (j < rows)
{
cout << "-"; // Display '-' to separate words
}
}
}
// Swap the values of b and w to change the sequence of words for the next row
t = b;
b = w;
w = t;
cout << endl; // Move to the next line after completing each row
}
}
Sample Output:
Display checkerboard pattern with the words 'black' and 'white': --------------------------------------------------------------------- Input number of rows: 5 black-white-black-white-black white-black-white-black-white black-white-black-white-black white-black-white-black-white black-white-black-white-black
Flowchart:
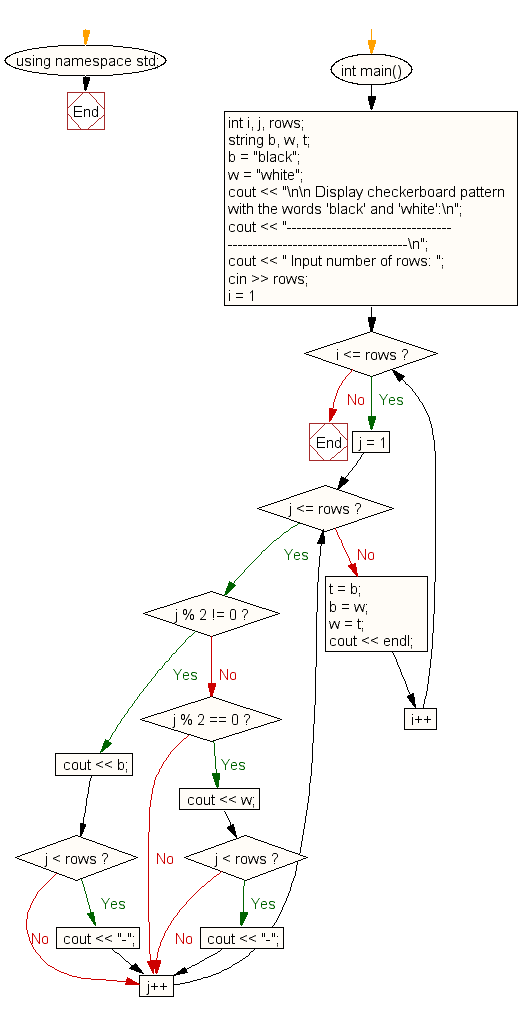
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find two's complement of a binary number.
Next: Write a program in C++ to calculate the sum of the series 1·2+2·3+3·4+4.5+5.6+.......
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-66.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics