C++ Exercises: Find two's complement of a binary number
C++ For Loop: Exercise-65 with Solution
Write a program in C++ to find the two's complement of a binary number.
Sample Solution:-
C++ Code :
#include <iostream> // Including the input/output stream library
#define SZ 8 // Defining a constant named SZ with a value of 8
using namespace std; // Using the standard namespace
int main() // Main function where the execution of the program starts
{
// Declare variables and character arrays to store binary values and their complements
char bn[SZ + 1], onComp[SZ + 1], twComp[SZ + 1];
int i, carr = 1; // Declare variables for iteration and carry initialization
int er = 0; // Initialize an error flag
// Display messages asking for input
cout << "\n\n Find two's complement of a binary value:\n";
cout << "----------------------------------------------\n";
cout << " Input a " << SZ << " bit binary value: ";
cin >> bn; // Read a binary value from the user
// Loop to find the one's complement of the entered binary value
for (i = 0; i < SZ; i++)
{
// Check each bit of the input binary number and create its one's complement
if (bn[i] == '1')
{
onComp[i] = '0';
}
else if (bn[i] == '0')
{
onComp[i] = '1';
}
else
{
cout << "Invalid Input. Input the value of assign bits." << endl; // Display an error message
er = 1; // Set error flag to 1
break; // Exit the loop
}
}
onComp[SZ] = '\0'; // Assign null terminator at the end of the one's complement array
// Loop to find the two's complement of the one's complement
for (i = SZ - 1; i >= 0; i--)
{
// Check each bit of the one's complement to calculate the two's complement
if (onComp[i] == '1' && carr == 1)
{
twComp[i] = '0';
}
else if (onComp[i] == '0' && carr == 1)
{
twComp[i] = '1';
carr = 0;
}
else
{
twComp[i] = onComp[i];
}
}
twComp[SZ] = '\0'; // Assign null terminator at the end of the two's complement array
// If no error occurred during input processing, display the original binary value, one's complement, and two's complement
if (er == 0)
{
cout << " The original binary = " << bn << endl;
cout << " After ones complement the value = " << onComp << endl;
cout << " After twos complement the value = " << twComp << endl;
}
}
Sample Output:
Find two's complement of a binary value: ---------------------------------------------- Input a 8 bit binary value: 01101110 The original binary = 01101110 After ones complement the value = 10010001 After twos complement the value = 10010010
Flowchart:
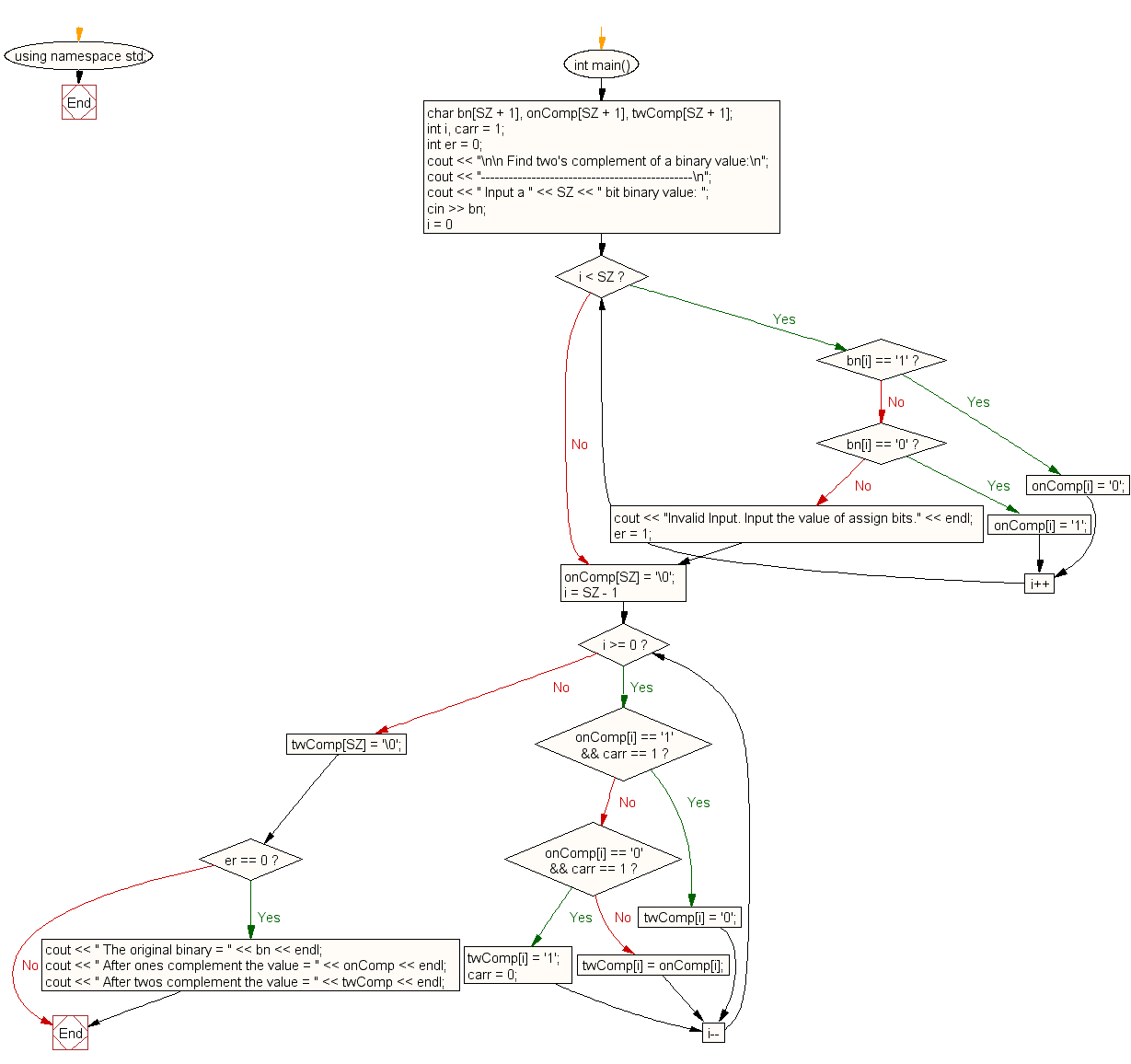
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find one's complement of a binary number.
Next: Write code to create a checkerboard pattern with the words "black" and "white".
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-65.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics