C++ Exercises: Find one's complement of a binary number
64. One's Complement of a Binary Number
Write a program in C++ to find the one's complement of a binary number.
Sample Solution:-
C++ Code :
#include <iostream> // Including the input/output stream library
#define SZ 8 // Defining a constant named SZ with a value of 8
using namespace std; // Using the standard namespace
int main() // Main function where the execution of the program starts
{
int i; // Declare an integer variable 'i'
char binary[SZ + 1], onesComp[SZ + 1]; // Declare character arrays to store binary values and their one's complement
int error = 0; // Initialize an error flag
// Display messages asking for input
cout << "\n\n Find one's complement of a binary value:\n";
cout << "----------------------------------------------\n";
cout << " Input a " << SZ << " bit binary value: ";
cin >> binary; // Read a binary value from the user
// Loop to find the one's complement of the entered binary value
for (i = 0; i < SZ; i++)
{
if (binary[i] == '1') // Check if the current bit is '1'
{
onesComp[i] = '0'; // If '1', replace it with '0' in the one's complement
}
else if (binary[i] == '0') // Check if the current bit is '0'
{
onesComp[i] = '1'; // If '0', replace it with '1' in the one's complement
}
else // If the input value contains any other character than '0' or '1'
{
cout << "Invalid Input. Input the value of assign bits." << endl; // Display an error message
error = 1; // Set error flag to 1
break; // Exit the loop
}
}
onesComp[SZ] = '\0'; // Assign null terminator at the end of the one's complement array
if (error == 0) // If no error occurred during input processing
{
// Display the original binary value and its one's complement
cout << " The original binary = " << binary << endl;
cout << " After ones complement the number = " << onesComp << endl;
}
}
Sample Output:
Find one's complement of a binary value: ---------------------------------------------- Input a 8 bit binary value: 10100101 The original binary = 10100101 After ones complement the number = 01011010
Flowchart:
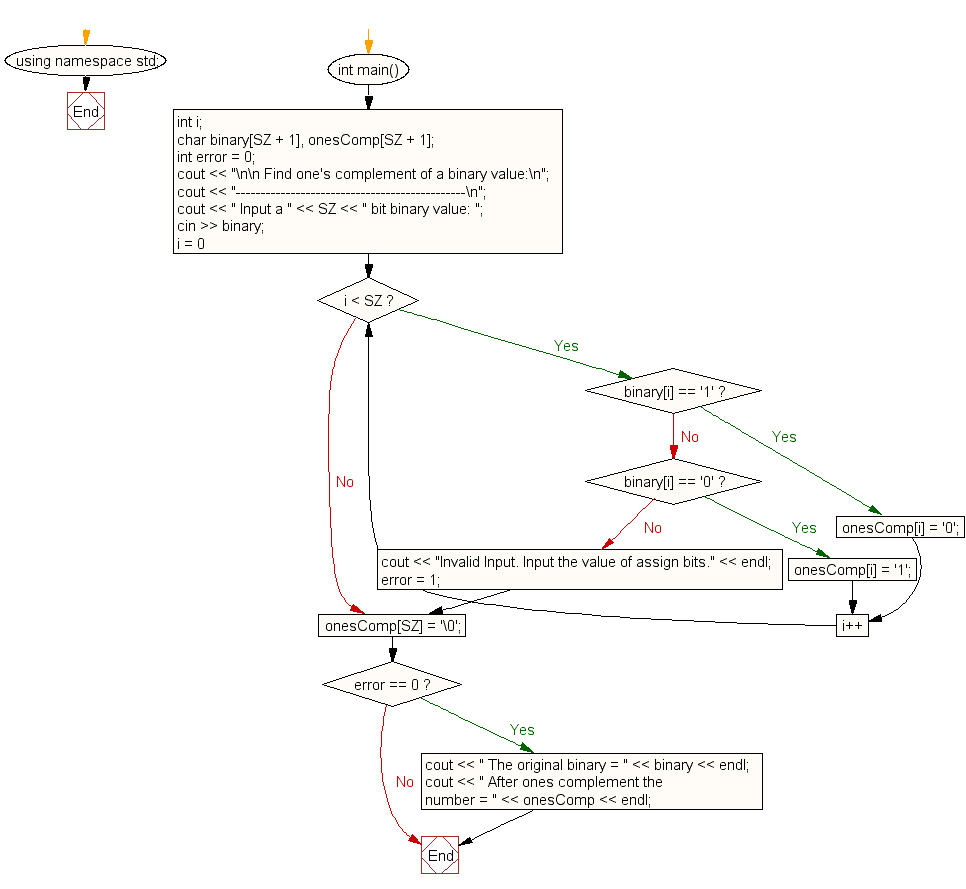
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the one's complement of an 8-bit binary number provided as input.
- Write a C++ program that reads a binary string, inverts each bit, and prints the resulting one's complement.
- Write a C++ program to convert an 8-bit binary number to its one's complement using character manipulation.
- Write a C++ program that processes an 8-bit binary input and outputs the one's complement by flipping each bit.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to enter any number and print all factors of the number.
Next: Write a program in C++ to find two's complement of a binary number.
What is the difficulty level of this exercise?