C++ Exercises: Print all factors of the number
63. Print All Factors of a Number
Write a program in C++ to enter any number and print all factors of the number.
Visual Presentation:
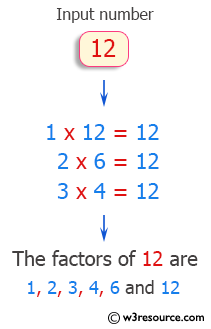
Sample Solution:-
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
int main() // Main function where the execution of the program starts
{
int num, i; // Declare integer variables 'num' and 'i'
// Display messages asking for input
cout << "\n\n Print all factors of a number:\n";
cout << "-----------------------------------\n";
cout << " Input a number: ";
cin >> num; // Read a number from the user
cout << "The factors are: "; // Display a message before listing factors
// Loop to find factors of the entered number
for (i = 1; i <= num; i++)
{
if (num % i == 0) // Check if 'i' divides 'num' completely (i.e., remainder is zero)
{
cout << i << " "; // If 'i' is a factor, display it
}
}
cout << endl; // Move to the next line after displaying all factors
}
Sample Output:
Print all factors of a number: ----------------------------------- Input a number: 63 The factors are: 1 3 7 9 21 63
Flowchart:
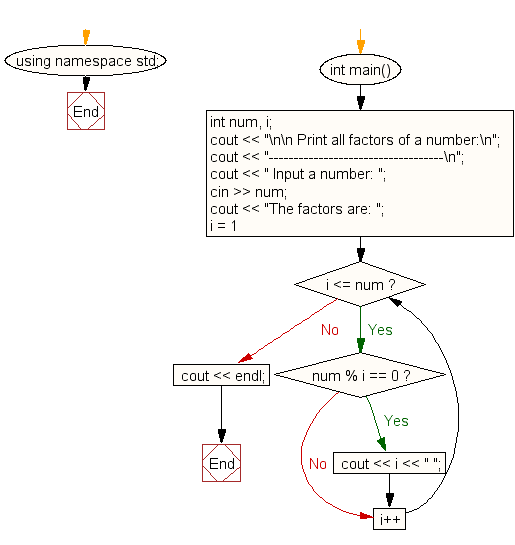
For more Practice: Solve these Related Problems:
- Write a C++ program to list all factors of a given number using a loop and conditional checks.
- Write a C++ program that reads a number and prints its factors in ascending order.
- Write a C++ program to determine and output all divisors of an integer by checking divisibility up to the number.
- Write a C++ program that calculates and displays all factors of a number using a for loop and modulus operator.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find power of any number using for loop.
Next: Write a program in C++ to find one's complement of a binary number.
What is the difficulty level of this exercise?