C++ Exercises: Find power of any number using for loop
62. Power Calculation Using a For Loop
Write a program in C++ to find the power of any number using a for loop.
Visual Presentation:
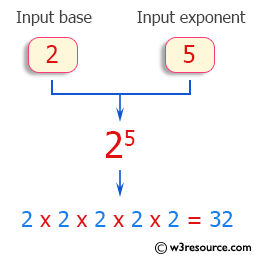
Sample Solution:-
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
int main() // Main function where the execution of the program starts
{
int bs, ex, num = 1, i; // Declare integer variables bs, ex, num, and i
// Display messages asking for input
cout << "\n\n Find power of any number using for loop:\n";
cout << "---------------------------------------------\n";
cout << " Input the base: ";
cin >> bs; // Read the base value from the user
cout << " Input the exponent: ";
cin >> ex; // Read the exponent value from the user
// Loop to calculate the power of the base number
for (i = 1; i <= ex; i++)
{
num = num * bs; // Multiply num by base 'ex' times to get the power
}
// Display the result of base raised to the exponent
cout << bs << " ^ " << ex << " = " << num << endl;
}
Sample Output:
Find power of any number using for loop: --------------------------------------------- Input the base: 2 Input the exponent: 5 2 ^ 5 = 32
Flowchart:
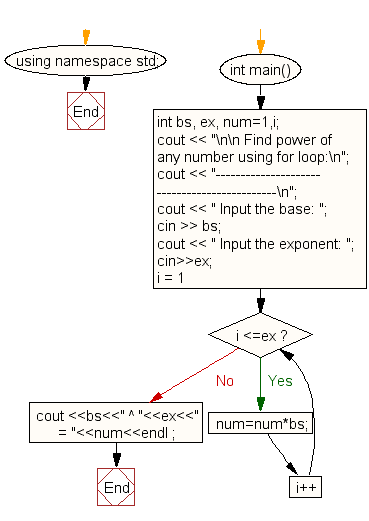
For more Practice: Solve these Related Problems:
- Write a C++ program to compute a number raised to a power using a for loop and iterative multiplication.
- Write a C++ program that reads a base and an exponent, then calculates the power by multiplying in a loop.
- Write a C++ program to calculate the power of a number using a for loop and display the computation step by step.
- Write a C++ program that implements exponentiation by iteratively multiplying the base, and prints the final result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to print all ASCII character with their values.
Next: Write a program in C++ to enter any number and print all factors of the number.
What is the difficulty level of this exercise?