C++ Exercises: Print all ASCII character with their values
Write a C++ program that prints all ASCII characters with their values.
Visual Presentation:
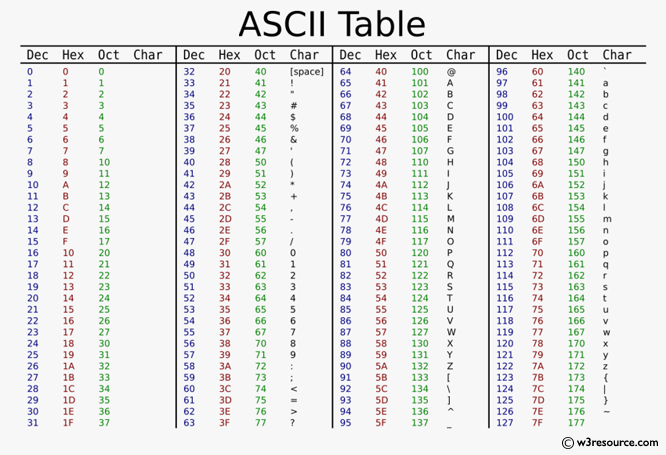
Sample Solution:-
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int sn, en, i, j, ctr, r; // Declare integer variables sn, en, i, j, ctr, and r
// Display messages asking for input
cout << "\n\n Print ASCII character with their values:\n";
cout << "-------------------------------------------------\n";
cout << " Input the starting value for ASCII characters: ";
cin >> sn; // Read starting value for ASCII characters from the user
cout << " Input the ending value for ASCII characters: ";
cin >> en; // Read ending value for ASCII characters from the user
// Checking if the starting or ending values are out of range (0-255)
if (sn < 255 || sn < 0)
sn = 1; // If starting value is out of range, set it to 1 (minimum ASCII value)
if (en < 0 || en > 255)
en = 255; // If ending value is out of range, set it to 255 (maximum ASCII value)
cout << "The ASCII characters:" << endl; // Output message
// Loop to print ASCII characters and their values from starting to ending value
for (i = sn; i <= en; i++)
{
cout << i << " --> " << char(i) << endl; // Print ASCII value and its corresponding character
}
}
Sample Output:
Print ASCII character with their values: ------------------------------------------------- Input the starting value for ASCII characters: 65 Input the ending value for ASCII characters: 75 The ASCII characters: 65 --> A 66 --> B 67 --> C 68 --> D 69 --> E 70 --> F 71 --> G 72 --> H 73 --> I 74 --> J 75 --> K
Flowchart:
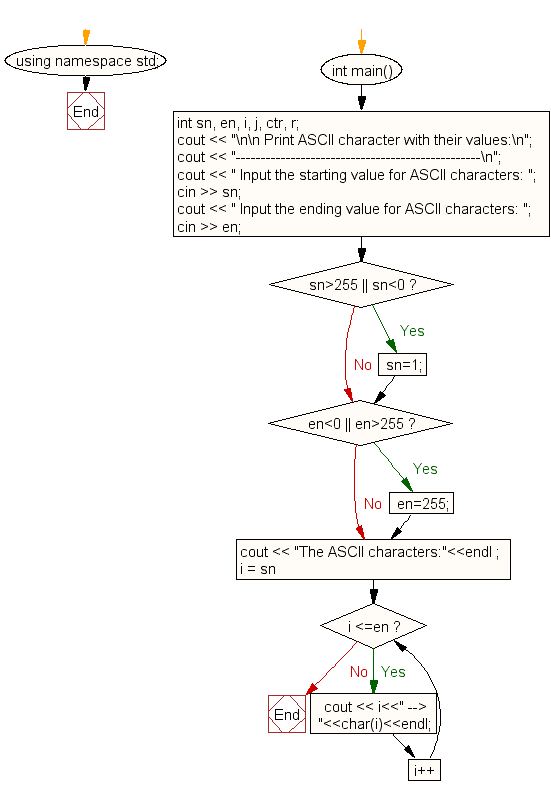
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to input any number and print it in words.
Next: Write a program in C++ to find power of any number using for loop.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics