C++ Exercises: Find prime number within a range
6. Find Prime Numbers Within a Range
Write a program in C++ to find a prime number within a range.
Sample Solution:
C++ Code:
#include <iostream> // Preprocessor directive to include the input/output stream header file
#include <math.h> // Preprocessor directive to include the math functions header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int num1, num2; // Declaration of integer variables 'num1', 'num2'
int fnd = 0, ctr = 0; // Initialization of integer variables 'fnd' and 'ctr' to zero
cout << "\n\n Find prime number within a range:\n"; // Display a message indicating the purpose
cout << "--------------------------------------\n"; // Display a separator line
cout << " Input number for starting range: "; // Prompting the user to input the starting range
cin >> num1; // Reading the starting range entered by the user
cout << " Input number for ending range: "; // Prompting the user to input the ending range
cin >> num2; // Reading the ending range entered by the user
cout << "\n The prime numbers between " << num1 << " and " << num2 << " are:" << endl; // Display a message indicating the range of numbers
for (int i = num1; i <= num2; i++) // Loop through each number within the given range
{
for (int j = 2; j <= sqrt(i); j++) // Loop to check for prime numbers
{
if (i % j == 0) // Checking if the number is divisible by 'j'
ctr++; // Incrementing 'ctr' if 'i' is divisible by 'j'
}
if (ctr == 0 && i != 1) // Checking if 'ctr' is zero and 'i' is not equal to 1 (prime numbers should not be 1)
{
fnd++; // Incrementing 'fnd' as a prime number is found
cout << i << " "; // Display the prime number
}
ctr = 0; // Resetting 'ctr' to zero for the next iteration
}
cout << "\n\n The total number of prime numbers between " << num1 << " to " << num2 << " is: " << fnd << endl; // Display the total count of prime numbers found
return 0; // Indicating successful completion of the program
}
Sample Output:
Find prime number within a range: -------------------------------------- Input number for starting range: 1 Input number for ending range: 100 The prime numbers between 1 and 100 are: 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97 The total number of prime numbers between 1 to 100 is: 25
Flowchart:
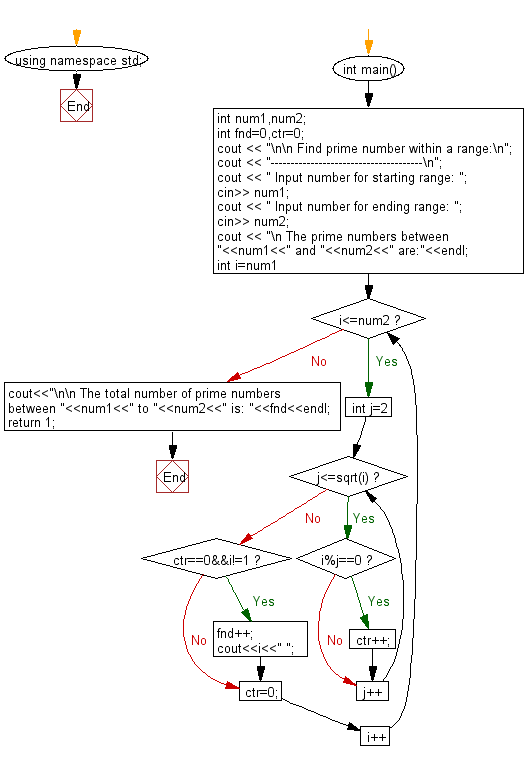
For more Practice: Solve these Related Problems:
- Write a C++ program to list all prime numbers between two input limits using a nested loop.
- Write a C++ program that prints prime numbers within a range and counts their total number.
- Write a C++ program to check each number in a given range for primality and display the prime numbers in a formatted list.
- Write a C++ program to find and display all primes between lower and upper bounds, using an efficient method for prime checking.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is prime or not.
Next: Write a program in C++ to find the factorial of a number.
What is the difficulty level of this exercise?