C++ Exercises: Find the sum of first and last digit of a number
57. Sum of First and Last Digits of a Number
Write a program in C++ to find the sum of the first and last digits of a number.
Visual Presentation:
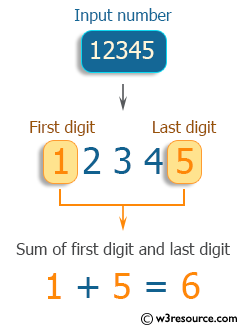
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int n, first, last, sum; // Declare integer variables n, first, last, and sum
// Display message asking for input
cout << "\n\n Find the sum of first and last digit of a number:\n";
cout << "------------------------------------------------------\n";
cout << " Input any number: ";
cin >> n; // Read input for the number from the user
first = n; // Assign the input number to the variable 'first'
last = n % 10; // Calculate the last digit of the number
for (first = n; first <= 10; first = first / 10); // Loop to find the first digit of the number
// Display the first and last digit of the number
cout << " The first digit of " << n << " is: " << first << endl;
cout << " The last digit of " << n << " is: " << last << endl;
// Calculate and display the sum of the first and last digit of the number
cout << " The sum of first and last digit of " << n << " is: " << first + last << endl;
}
Sample Output:
Find the sum of first and last digit of a number: ------------------------------------------------------ Input any number: 12345 The first digit of 12345 is: 1 The last digit of 12345 is: 5 The sum of first and last digit of 12345 is: 6
Flowchart:
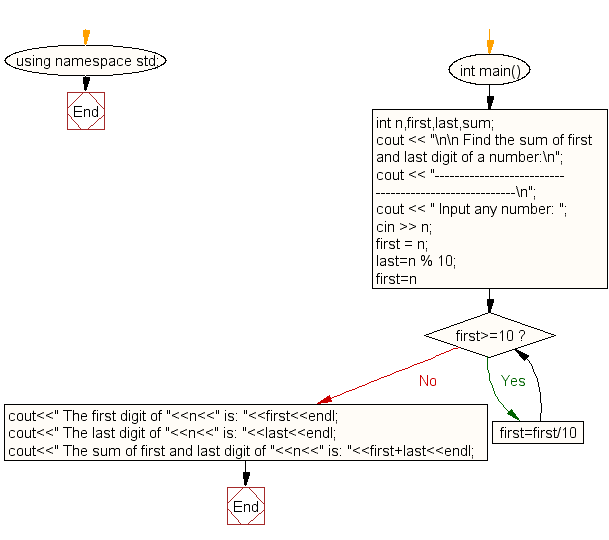
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the sum of the first and last digits of an integer by extracting digits using division and modulus.
- Write a C++ program that reads a number and prints the sum of its first and last digits computed without converting the number to a string.
- Write a C++ program to obtain the first digit by iterating until the number is less than 10, and add it to the last digit.
- Write a C++ program that computes and displays the sum of the boundary digits of an input number using arithmetic operations.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the first and last digit of a number.
Next: Write a program in C++ to calculate product of digits of any number.
What is the difficulty level of this exercise?