C++ Exercises: Find the first and last digit of a number
56. First and Last Digits of a Number
Write a program in C++ to find the first and last digits of a number.
Visual Presentation:

Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int n, first, last; // Declare integer variables n, first, and last
// Display message asking for input
cout << "\n\n Find the first and last digit of a number:\n";
cout << "-----------------------------------------------\n";
cout << " Input any number: ";
cin >> n; // Read input for the number from the user
first = n; // Assign the input number to the variable 'first'
last = n % 10; // Calculate the last digit of the number
for (first = n; first >= 10; first = first / 10); // Loop to find the first digit of the number
// Display the first and last digit of the number
cout << " The first digit of " << n << " is: " << first << endl;
cout << " The last digit of " << n << " is: " << last << endl;
}
Sample Output:
Find the first and last digit of a number: ----------------------------------------------- Input any number: 5679 The first digit of 5679 is: 5 The last digit of 5679 is: 9
Flowchart:
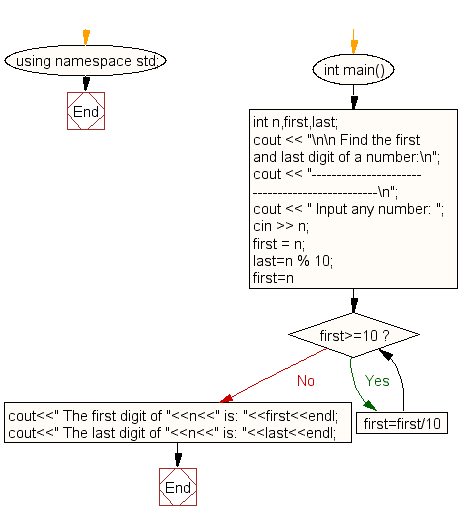
56. First and Last Digits of a Number
For more Practice: Solve these Related Problems:
- Write a C++ program to extract and print the first and last digits of an input number using loops.
- Write a C++ program that converts a number to a string and prints the first and last characters as digits.
- Write a C++ program to find the first digit by repeatedly dividing the number and the last digit using modulus.
- Write a C++ program that reads an integer and outputs its first and last digits, using both arithmetic and string conversion methods.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display such a pattern for n number of rows using number. Each row will contain odd numbers of number.
The first and last number of each row will be 1 and middle column will be the row number. n numbers of columns will appear in 1st row.
Next: Write a program in C++ to find the sum of first and last digit of a number.
What is the difficulty level of this exercise?