C++ Exercises: Display specified pattern for n number of rows using number
C++ For Loop: Exercise-55 with Solution
Write a program in C++ to display such a pattern for n number of rows using numbers. There will be odd numbers in each row. The first and last number of each row will be 1 and the middle column will be the row number. N numbers of columns will appear in the 1st row.
Input number of rows: 7 1234567654321 12345654321 123454321 1234321 12321 121 1
Visual Presentation:
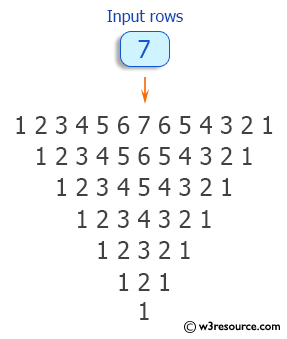
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, n; // Declare integer variables i, j, and n
// Display message asking for input
cout << "\n\n Display a pattern using odd number of numbers, the n numbers of columns will appear in 1st row:\n";
cout << "----------------------------------------------------------------------------------------------------\n";
cout << " Input number of rows: ";
cin >> n; // Read input for the number of rows from the user
for (i = n; i <= 1; i--) // Loop for the number of rows in reverse order
{
/* print blank spaces based on the row number */
for (j = 1; j <= n + 5 - i; j++) // Print spaces based on row number for formatting
cout << " "; // Print spaces
/* Display numbers in ascending order up to the middle */
for (j = 1; j <= i; j++) // Loop to print numbers in ascending order
cout << j; // Print the numbers in ascending order
/* Display numbers in reverse order after the middle */
for (j = i - 1; j >= 1; j--) // Loop to print numbers in reverse order after the middle
cout << j; // Print the numbers in reverse order
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display a pattern using odd number of numbers, the n numbers of column s will appear in 1st row: ----------------------------------------------------------------------- ----------------------------- Input number of rows: 7 1234567654321 12345654321 123454321 1234321 12321 121 1
Flowchart:
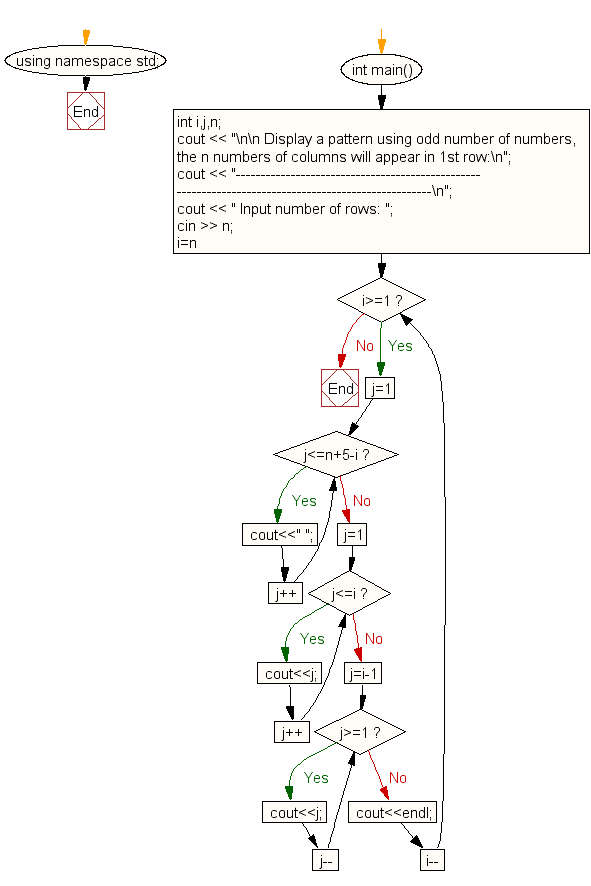
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern powerof2triangle.
Next: Write a program in C++ to find the first and last digit of a number.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-55.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics