C++ Exercises: Display the pattern using digits with left justified and the highest columns appears in first row in descending order
52. Left-Justified Descending Pattern
Write a C++ program to display the pattern using digits with left justified spacing and the highest columns appearing in the first row in descending order.
Visual Presentation:
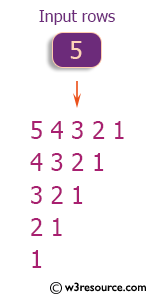
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, rows, d; // Declare integer variables i, j, rows, and d
// Display message asking for input
cout << "\n\n Display the pattern using digits with left justified:\n";
cout << "----------------------------------------------------------\n";
cout << " Input number of rows: ";
cin >> rows; // Read input for the number of rows from the user
d = 0; // Initialize variable d to 0
for (i = 1; i <= rows; i++) // Loop for the number of rows
{
for (j = rows - d; j >= 1; j--) // Loop to print digits in descending order
{
cout << j << " "; // Print the digit followed by a space
}
d++; // Increment d for each row
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the pattern using digits with left justified: ---------------------------------------------------------- Input number of rows: 5 5 4 3 2 1 4 3 2 1 3 2 1 2 1 1
Flowchart:
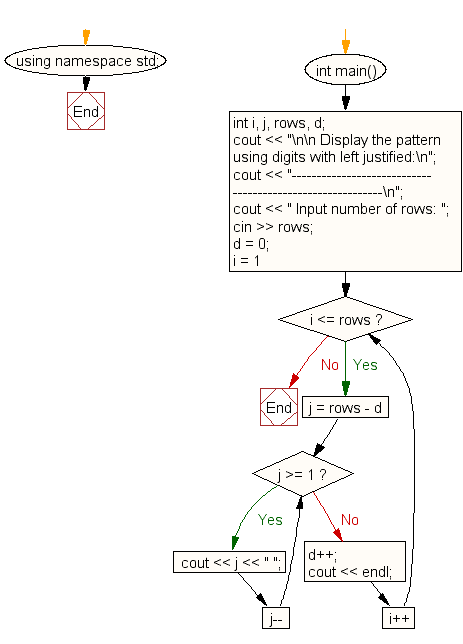
For more Practice: Solve these Related Problems:
- Write a C++ program to display a left-justified pattern where the first row shows numbers in descending order from n to 1.
- Write a C++ program that prints rows of numbers starting with n decreasing down to 1, with each row left aligned.
- Write a C++ program to output a pattern of descending numbers where the first row is complete and each subsequent row loses one digit from the start.
- Write a C++ program to generate a left-justified numerical pattern that starts with n digits and reduces by one digit per row.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern using digits with right justified and the highest columns appears in first row.
Next: Write a program in C++ to display the pattern like right angle triangle with right justified using digits.
What is the difficulty level of this exercise?