C++ Exercises: Print a pattern like highest numbers of columns appear in first row
50. Descending Column Pattern
Write a C++ program to print a pattern in which the highest number of columns appears in the first row.
Visual Presentation:
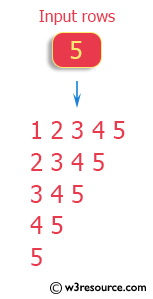
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, n; // Declare integer variables i, j, and n
// Display message asking for input
cout << "\n\n Display the pattern like highest numbers of columns appear in first row:\n";
cout << "------------------------------------------------------------------------------\n";
cout << " Input the number of rows: ";
cin >> n; // Read input for the number of rows from the user
for (i = 1; i <= n;) // Loop for the number of rows
{
cout << i; // Print the current value of 'i'
for (j = i + 1; j <= n;) // Loop to print the numbers after 'i'
{
cout << j; // Print the number
j = j + 1; // Increment 'j'
}
cout << endl; // Move to the next line after each row is printed
i = i + 1; // Increment 'i' for the next row
}
}
Sample Output:
Display the pattern like highest numbers of columns appear in first row: ------------------------------------------------------------------------------ Input the number of rows: 5 12345 2345 345 45 5
Flowchart:
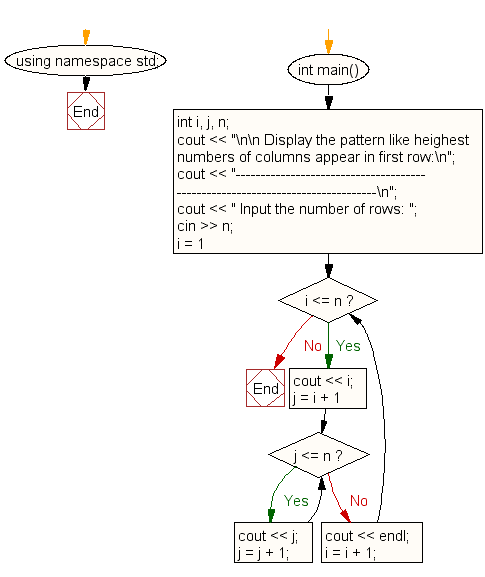
For more Practice: Solve these Related Problems:
- Write a C++ program to print a pattern where the first row contains numbers from 1 to n and each subsequent row omits the first digit.
- Write a C++ program that displays a descending column pattern by printing the first row fully and then reducing one number per row from the beginning.
- Write a C++ program to create a pattern in which the top row has n columns and each next row starts with the next integer in sequence.
- Write a C++ program that reads an integer and outputs a pattern where each row has one fewer element than the previous row, starting from 1 to n.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to print a pyramid of digits as shown below for n number of lines.
Next: Write a program in C++ to display the pattern using digits with right justified and the highest columns appears in first row.
What is the difficulty level of this exercise?