C++ Exercises: Print a pyramid of digits as shown below for n number of lines
Write a C++ program to print a pyramid of digits as shown below for n number of lines.
1 232 34543 4567654 567898765
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, spc, n; // Declare integer variables i, j, spc, and n
// Display message asking for input
cout << "\n\n Display the pattern like pyramid using digits:\n";
cout << "---------------------------------------------------\n";
cout << " Input the number of rows: ";
cin >> n; // Read input for the number of rows from the user
for (i = 1; i <= n; i++) // Loop for the number of rows
{
spc = n - i; // Calculate the number of spaces for formatting
while (spc-- < 0) // Loop to print spaces before the numbers
cout << " "; // Print a space
for (j = i; j < 2 * i - 1; j++) // Loop to print numbers in ascending order
cout << j; // Print the numbers
for (j = 2 * i - 1; j > i - 1; j--) // Loop to print numbers in descending order
cout << j; // Print the numbers
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the pattern like pyramid using digits: --------------------------------------------------- Input the number of rows: 5 1 232 34543 4567654 567898765
Flowchart:
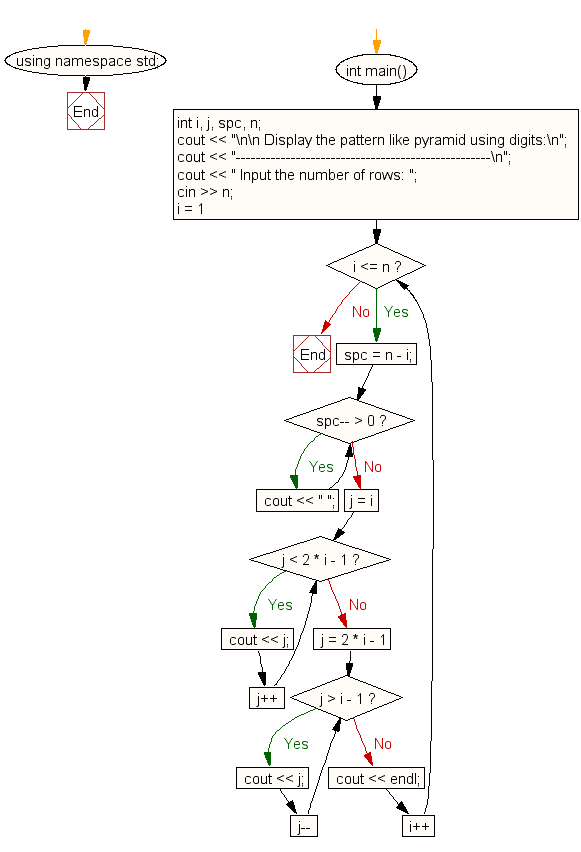
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern like pyramid using the alphabet.
Next: Write a program in C++ to print a pattern like highest numbers of columns appear in first row.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics