C++ Exercises: Display the pattern like pyramid using the alphabet
48. Alphabet Pyramid
Write a program in C++ to display the pyramid pattern using the alphabet.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j; // Declare integer variables i and j
char alph = 'A'; // Declare and initialize a character variable alph with 'A'
int n, blk; // Declare integer variables n and blk
int ctr = 1; // Initialize an integer variable ctr with value 1
// Display message asking for input
cout << "\n\n Display the pattern like pyramid using the alphabet:\n";
cout << "---------------------------------------------------------\n";
cout << " Input the number of Letters (less than 26) in the Pyramid: ";
cin >> n; // Read input for the number of letters from the user
for (i = 1; i <= n; i++) // Loop for the number of rows
{
for (blk = 1; blk <= n - i; blk++) // Loop to print spaces before the characters
cout << " "; // Print two spaces for formatting
for (j = 0; j <= (ctr / 2); j++) // Loop to print characters in ascending order
{
cout << alph++ << " "; // Print the character and increment the value of alph
}
alph = alph - 2; // Decrement alph by 2 to get the previous character
for (j = 0; j > (ctr / 2); j++) // Loop to print characters in descending order
{
cout << alph-- << " "; // Print the character and decrement the value of alph
}
ctr = ctr + 2; // Increment ctr by 2 for the next row
alph = 'A'; // Reset alph to 'A' for the next row
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the pattern like pyramid using the alphabet: --------------------------------------------------------- Input the number of Letters (less than 26) in the Pyramid: 5 A A B A A B C B A A B C D C B A A B C D E D C B A
Flowchart:
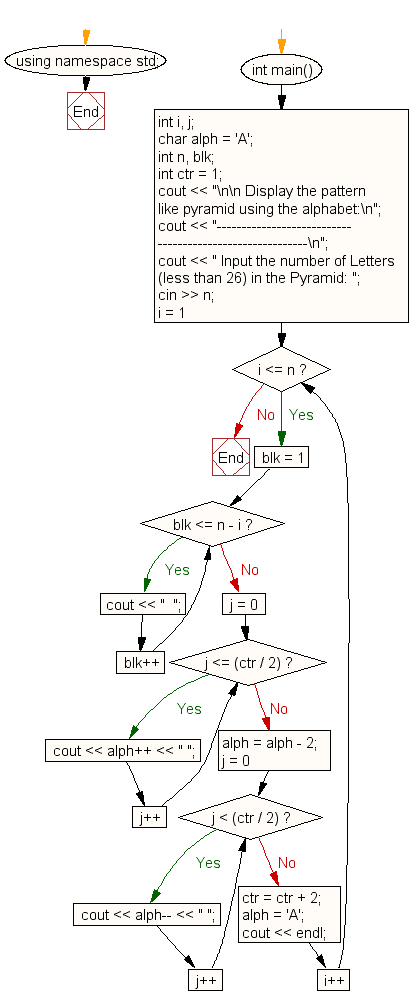
For more Practice: Solve these Related Problems:
- Write a C++ program to print a pyramid pattern using the alphabet, where each row expands symmetrically with letters.
- Write a C++ program that reads an integer and displays an alphabet pyramid with letters incrementing up to the given number.
- Write a C++ program to generate a centered pyramid of letters where each row shows letters in sequence from A up to a specific character and then in reverse.
- Write a C++ program to output a pyramid pattern of the alphabet with spaces to align each row centrally.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display such a pattern for n number of rows using number. Each row will contain odd numbers of number.
The first and last number of each row will be 1 and middle column will be the row number.
Next: Write a program in C++ to print a pyramid of digits as shown below for n number of lines.
What is the difficulty level of this exercise?