C++ Exercises: Print the Floyd's Triangle
Write a C++ program to print Floyd's Triangle.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, n, p, q; // Declare integer variables i, j, n, p, and q
// Display message asking for input
cout << "\n\n Print the Floyd's Triangle:\n";
cout << "--------------------------------\n";
cout << " Input number of rows: ";
cin >> n; // Read input for the number of rows from the user
// Loop to print Floyd's Triangle pattern with alternating 0 and 1
for (i = 1; i <= n; i++) // Loop for the number of rows
{
if (i % 2 == 0) // Check if the current row number is even
{
p = 1; // Assign 1 to 'p' if the row number is even
q = 0; // Assign 0 to 'q' if the row number is even
}
else // If the current row number is odd
{
p = 0; // Assign 0 to 'p' if the row number is odd
q = 1; // Assign 1 to 'q' if the row number is odd
}
for (j = 1; j <= i; j++) // Loop to print numbers in each row
{
if (j % 2 == 0) // Check if the current position in the row is even
cout << p; // Print 'p' if the position is even
else
cout << q; // Print 'q' if the position is odd
}
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Print the Floyd's Triangle: -------------------------------- Input number of rows: 5 1 01 101 0101 10101
Flowchart:
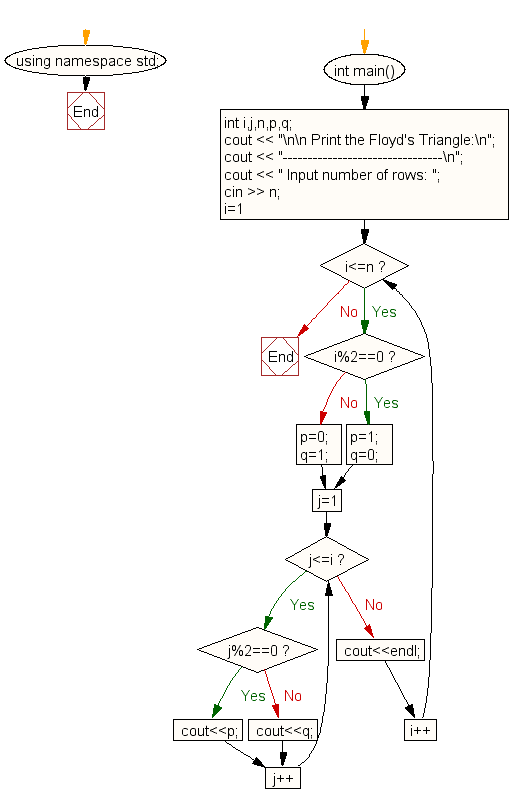
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern like a pyramid using asterisk and each row contain an odd number of asterisks.
Next: Write a program in C++ to display the pattern like a diamond.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics