C++ Exercises: Display the pattern like a pyramid with an asterisk
Write a C++ program to make such a pattern like a pyramid with an asterisk.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
#include <string> // Include the string handling library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, spc, rows, k; // Declare integer variables i, j, spc, rows, and k
// Display message asking for input
cout << "\n\n Display such a pattern like a pyramid with an asterisk:\n";
cout << "------------------------------------------------------------\n";
cout << " Input number of rows: ";
cin >> rows; // Read input for the number of rows from the user
spc = rows + 4 - 1; // Calculate the number of spaces for formatting
// Loop to print the pyramid pattern with asterisks
for (i = 1; i <= rows; i++) // Loop for the number of rows
{
for (k = spc; k >= 1; k--) // Loop to print spaces before the asterisks
{
cout << " "; // Print a space
}
for (j = 1; j <= i; j++) // Loop to print asterisks in each row
{
cout << "* "; // Print an asterisk followed by a space
}
cout << endl; // Move to the next line after each row is printed
spc--; // Decrement the number of spaces for the next row
}
}
Sample Output:
Display such a pattern like a pyramid with an asterisk: ------------------------------------------------------------ Input number of rows: 5 * * * * * * * * * * * * * * *
Flowchart:
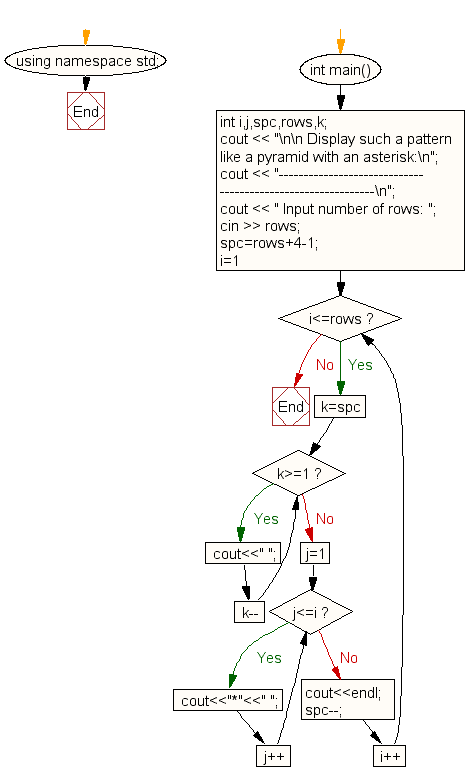
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to make such a pattern like a pyramid with numbers increased by 1.
Next: Write a program in C++ to make such a pattern like a pyramid using number and a number will repeat for a row.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics