C++ Exercises: Display the pattern like right angle triangle with number
36. Display Right-Angle Triangle Pattern with Numbers
Write a program in C++ to display the pattern like right angle triangle with number.
Visual Presentation:
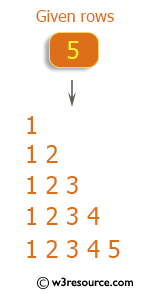
Sample Solution:-
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, rows; // Declare integer variables i, j, and rows
// Display message asking for input
cout << "\n\n Display the pattern using number starting from 1:\n";
cout << "------------------------------------------------------\n";
cout << " Input number of rows: ";
cin >> rows; // Read input for the number of rows from the user
// Loop to print the pattern using numbers starting from 1
for (i = 1; i <= rows; i++) // Loop for the number of rows
{
for (j = 1; j <= i; j++) // Nested loop for printing numbers in each row
{
cout << j; // Print the current value of 'j' (numbers starting from 1)
}
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the pattern using number starting from 1: ------------------------------------------------------ Input number of rows: 5 1 12 123 1234 12345
Flowchart:
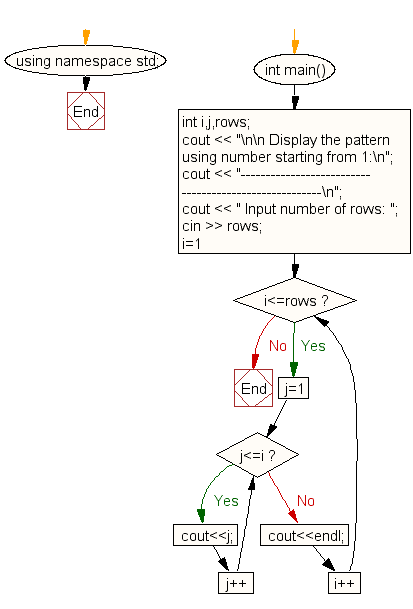
For more Practice: Solve these Related Problems:
- Write a C++ program to print a right-angled triangle pattern where each row contains sequential numbers starting from 1.
- Write a C++ program that displays a triangle with numbers incremented on each row using nested loops.
- Write a C++ program to output a numeric triangle pattern where the first row is "1", the second row is "12", and so on.
- Write a C++ program that reads the number of rows and prints a right-angle triangle pattern with concatenated numbers in each row.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern like right angle triangle using an asterisk.
Next: Write a program in C++ to make such a pattern like right angle triangle using number which will repeat the number for that row.
What is the difficulty level of this exercise?