C++ Exercises: Find out the sum of an A.P. series
Write a C++ program to find the sum of an A.P. series.
Visual Presentation:
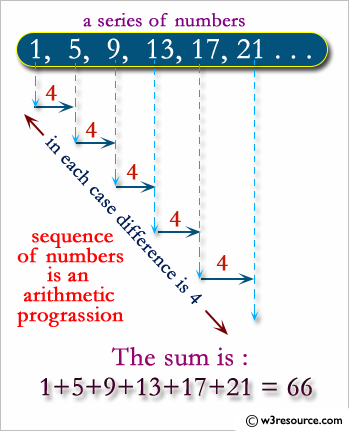
Sample Solution:-
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int n1, df, n2, i, ln; // Declare integer variables n1, df, n2, i, ln
int s1 = 0; // Declare and initialize an integer variable s1 to 0
// Display message asking for input
cout << "\n\n Find out the sum of A.P. series:\n";
cout << "-----------------------------------------\n";
cout << " Input the starting number of the A.P. series: ";
cin >> n1; // Read input for starting number of the A.P. series
cout << " Input the number of items for the A.P. series: ";
cin >> n2; // Read input for the number of items for the A.P. series
cout << " Input the common difference of A.P. series: ";
cin >> df; // Read input for the common difference of A.P. series
// Calculate the sum of the A.P. series using the formula
s1 = (n2 * (2 * n1 + (n2 - 1) * df)) / 2;
ln = n1 + (n2 - 1) * df; // Calculate the last number in the series
// Display the sum of the A.P. series
cout << " The Sum of the A.P. series are : " << endl;
// Loop through the A.P. series and display each term
for (i = n1; i <= ln; i = i + df)
{
if (i != ln)
cout << i << " + "; // Display series term followed by '+'
else
cout << i << " = " << s1 << endl; // Display last term followed by '=' and the calculated sum
}
}
Sample Output:
Find out the sum of A.P. series: ----------------------------------------- Input the starting number of the A.P. series: 1 Input the number of items for the A.P. series: 8 Input the common difference of A.P. series: 5 The Sum of the A.P. series are : 1 + 6 + 11 + 16 + 21 + 26 + 31 + 36 = 148
Flowchart:
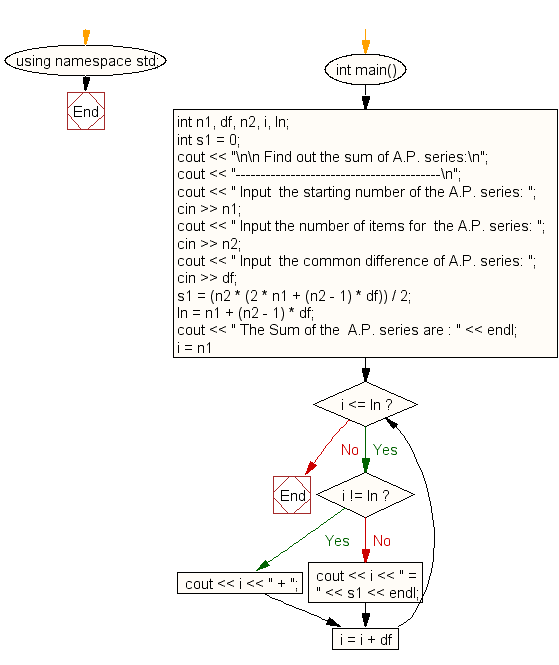
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the number in reverse order.
Next: Write a program in C++ to find the Sum of GP series.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics