C++ Exercises: Display the number in reverse order
30. Display the Number in Reverse Order
Write a program in C++ to display the numbers in reverse order.
Visual Presentation:
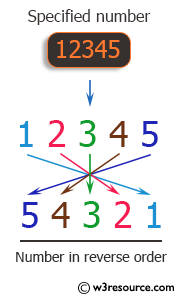
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int num, r, sum = 0, t; // Declaration of integer variables: 'num' (input number), 'r' (remainder), 'sum' (stores the reversed number), 't' (temporary variable)
cout << "\n\n Display the number in reverse order:\n"; // Displaying a message on the console
cout << "-----------------------------------------\n";
cout << " Input a number: "; // Asking the user for a number
cin >> num; // Reading the number from the user
for (t = num; num != 0; num = num / 10) // Reversing the number using a loop
{
r = num % 10; // Getting the rightmost digit of the number
sum = sum * 10 + r; // Constructing the reversed number by adding digits one by one from the original number
}
cout << " The number in reverse order is : " << sum << endl; // Displaying the reversed number
}
Sample Output:
Display the number in reverse order: ----------------------------------------- Input a number: 12345 The number in reverse order is : 54321
Flowchart:
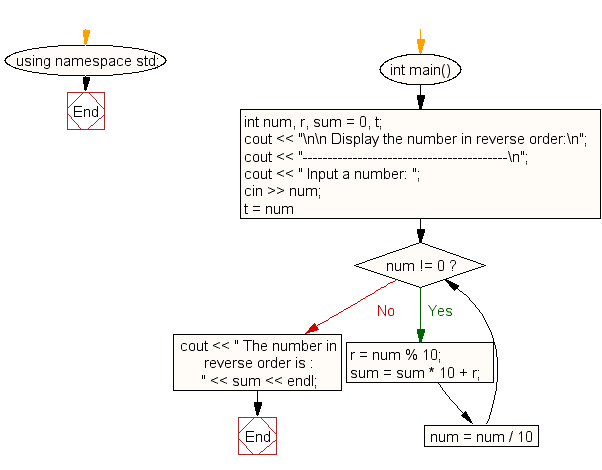
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse the digits of an input number using a while loop and modulus operations.
- Write a C++ program that converts a number to a string, reverses the string, and prints the reversed number.
- Write a C++ program to iterate over the digits of a number and construct its reverse without converting to string.
- Write a C++ program that reads a positive integer and outputs its digits in reverse order using recursion.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find LCM of any two numbers using HCF.
Next: Write a program in C++ to find out the sum of an A.P. series.
What is the difficulty level of this exercise?