C++ Exercises: Find LCM of any two numbers using HCF
29. Find the LCM of Two Numbers Using HCF
Write a program in C++ to find the LCM of any two numbers using HCF.
Visual Presentation:
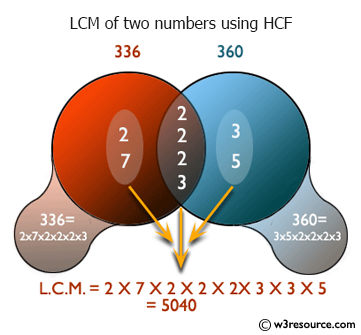
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int i, n1, n2, j, hcf = 1, lcm; // Declaration of integer variables: 'i' (loop counter), 'n1' and 'n2' (input numbers), 'j' (temporary variable), 'hcf' (stores the HCF), 'lcm' (stores the LCM)
cout << "\n\n LCM of two numbers:\n"; // Displaying a message on the console
cout << "------------------------\n";
cout << " Input 1st number for LCM: "; // Asking the user for the 1st number
cin >> n1; // Reading the 1st number from the user
cout << " Input 2nd number for LCM: "; // Asking the user for the 2nd number
cin >> n2; // Reading the 2nd number from the user
j = (n1 < n2) ? n1 : n2; // Determining the smaller of the two numbers
for (i = 1; i <= j; i++) { // Loop to find the Highest Common Factor (HCF)
if (n1 % i == 0 && n2 % i == 0) { // Checking if 'i' is a common factor of both numbers
hcf = i; // Updating the HCF when 'i' is a common factor
}
}
/* mltiplication of HCF and LCM = the multiplication of these two numbers.*/
lcm = (n1 * n2) / hcf; // Calculating the Least Common Multiple (LCM) using the formula LCM * HCF = Product of the numbers
cout << " The LCM of " << n1 << " and " << n2 << " is: " << lcm << endl; // Displaying the calculated LCM
}
Sample Output:
LCM of two numbers: ------------------------ Input 1st number for LCM: 15 Input 2nd number for LCM: 25 The LCM of 15 and 25 is: 75
Flowchart:
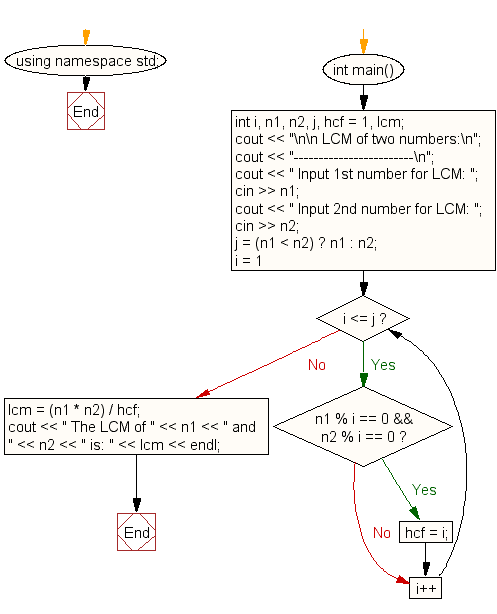
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the LCM of two numbers by first calculating their GCD using Euclid’s algorithm.
- Write a C++ program that reads two numbers and computes their least common multiple via the formula LCM = (a*b)/GCD(a, b).
- Write a C++ program to find the LCM of two integers using a while loop to search for the smallest common multiple.
- Write a C++ program that implements both recursive GCD and iterative LCM calculations for two input numbers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the number and sum of all integer between 100 and 200 which are divisible by 9.
Next: Write a program in C++ to display the number in reverse order.
What is the difficulty level of this exercise?