C++ Exercises: Display the first n terms of Fibonacci series
27. Display the First n Terms of the Fibonacci Series
Write a program in C++ to display the first n terms of the Fibonacci series.
Visual Presentation:
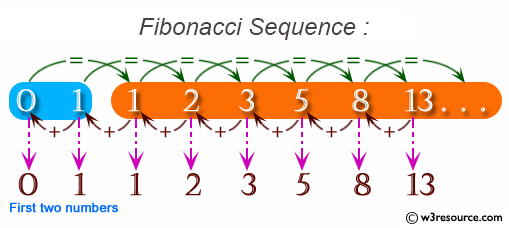
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int prv = 0, pre = 1, trm, i, n; // Declaration of integer variables 'prv' (previous term), 'pre' (current term), 'trm' (next term), 'i' (loop counter), 'n' (number of terms)
cout << "\n\n Display the first n terms of Fibonacci series:\n"; // Displaying a message on the console
cout << "---------------------------------------------------\n";
cout << " Input number of terms to display: "; // Prompting the user to input the number of terms
cin >> n; // Reading the input value as the number of terms
cout << " Here is the Fibonacci series up to " << n << " terms: " << endl; // Displaying a message indicating the number of terms for the Fibonacci series
cout << prv << " " << pre; // Displaying the first two terms of the Fibonacci series
for (i = 3; i <= n; i++) // Loop to generate and display the Fibonacci series from the third term
{
trm = prv + pre; // Calculating the next term of the Fibonacci series
cout << " " << trm; // Displaying the next term of the Fibonacci series
prv = pre; // Updating the previous term to the current term
pre = trm; // Updating the current term to the next term
}
cout << endl; // Printing a new line after displaying the series
}
Sample Output:
Display the first n terms of Fibonacci series: --------------------------------------------------- Input number of terms to display: 10 Here is the Fibonacci series upto to 10 terms: 0 1 1 2 3 5 8 13 21 34
Flowchart:
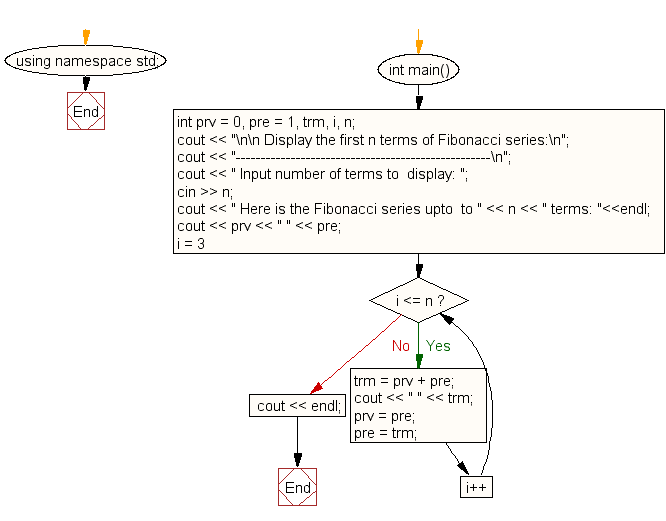
For more Practice: Solve these Related Problems:
- Write a C++ program to generate the Fibonacci sequence up to n terms using recursion.
- Write a C++ program that prints the Fibonacci series for a given n using iterative loops and stores the results in an array.
- Write a C++ program to display the Fibonacci series up to n terms and also print the nth Fibonacci number.
- Write a C++ program that uses a while loop to generate and display the first n numbers in the Fibonacci sequence.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
Next: Write a program in C++ to find the number and sum of all integer between 100 and 200 which are divisible by 9.
What is the difficulty level of this exercise?