C++ Exercises: Find the sum of the series 1 +11 + 111 + 1111 + .. n terms
26. Sum of the Series [1 + 11 + 111 + ...]
Write a program in C++ to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
Visual Presentation:
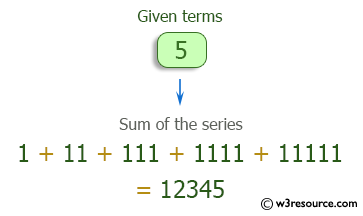
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int n, i, sum = 0; // Declaration of integer variables 'n' (number of terms), 'i' (loop counter), 'sum' (to store the sum)
int t = 1; // Initializing variable 't' to 1
cout << "\n\n Display the sum of the series 1 + 11 + 111 + 1111 + .. n terms:\n"; // Displaying a message on the console
cout << "-------------------------------------------------------------------\n";
cout << " Input number of terms: "; // Prompting the user to input the number of terms
cin >> n; // Reading the input value as the number of terms
for (i = 1; i <= n; i++) // Loop to generate the series and calculate the sum
{
cout << t << " "; // Displaying the current term of the series
if (i < n) // Checking if it's not the last term to print '+'
{
cout << "+ "; // Displaying '+' to separate terms if it's not the last term
}
sum = sum + t; // Adding the current term to the sum
t = (t * 10) + 1; // Generating the next term of the series by appending '1' to the current term
}
cout << "\n The sum of the series is: " << sum << endl; // Displaying the final sum of the series
}
Sample Output:
Display the sum of the series 1 +11 + 111 + 1111 + .. n terms: ------------------------------------------------------------------- Input number of terms: 5 1 + 11 + 111 + 1111 + 11111 The sum of the series is: 12345
Flowchart:
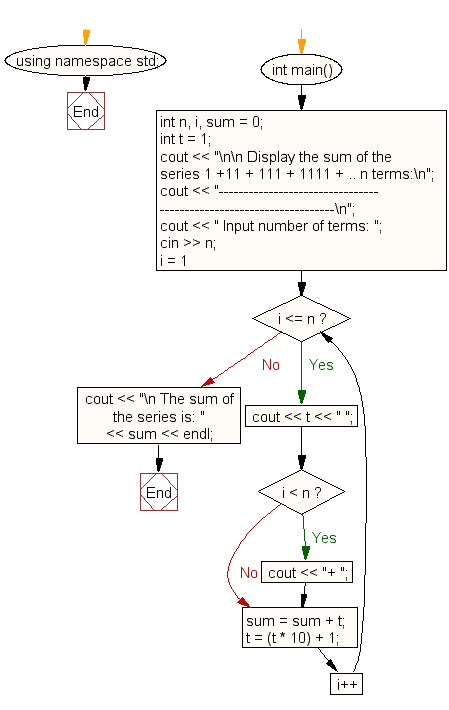
For more Practice: Solve these Related Problems:
- Write a C++ program to generate a series where each term is formed by repeating the digit 1, and compute the sum for n terms.
- Write a C++ program that reads a number n and prints the series 1, 11, 111, ... up to n terms, then outputs the total sum.
- Write a C++ program to construct numbers made entirely of 1's with increasing lengths and add them together.
- Write a C++ program that forms the series of repeated 1's for n terms and calculates their cumulative sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of the series [ x - x^3 + x^5 + ......].
Next: Write a program in C++ to display the first n terms of Fibonacci series.
What is the difficulty level of this exercise?