C++ Exercises: Display the n terms of harmonic series and their sum
22. Display n Terms of a Harmonic Series and Their Sum
Write a program in C++ to display the n terms of a harmonic series and their sum.
1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n terms.
Visual Presentation:
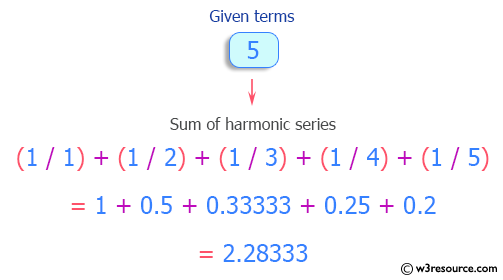
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int i, n; // Declaration of integer variables 'i' (loop counter) and 'n' (user input for the number of terms)
float s = 0.0; // Declaration of a floating-point variable 's' initialized to 0.0 (sum of the harmonic series)
cout << "\n\n Display n terms of harmonic series and their sum:\n"; // Displaying a message on the console
cout << " The harmonic series: 1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n terms\n"; // Displaying information about the harmonic series
cout << "-----------------------------------------------------------------\n";
cout << " Input number of terms: "; // Prompting the user to input the number of terms
cin >> n; // Reading the input value as the number of terms
for (i = 1; i <= n; i++) // Loop for 'n' terms
{
if (i < n) // Checking if 'i' is less than 'n'
{
cout << "1/" << i << " + "; // Displaying the term of the series
s += 1 / (float)i; // Adding the reciprocal of 'i' to the sum 's' (converting 'i' to float for accurate division)
}
if (i == n) // Checking if 'i' is equal to 'n'
{
cout << "1/" << i; // Displaying the last term of the series
s += 1 / (float)i; // Adding the reciprocal of 'i' to the sum 's' (converting 'i' to float for accurate division)
}
}
cout << "\n The sum of the series up to " << n << " terms: " << s << endl; // Displaying the sum of the harmonic series up to 'n' terms
}
Sample Output:
Display n terms of harmonic series and their sum: The harmonic series: 1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n terms ----------------------------------------------------------------- Input number of terms: 5 1/1 + 1/2 + 1/3 + 1/4 + 1/5 The sum of the series upto 5 terms: 2.28333
Flowchart:
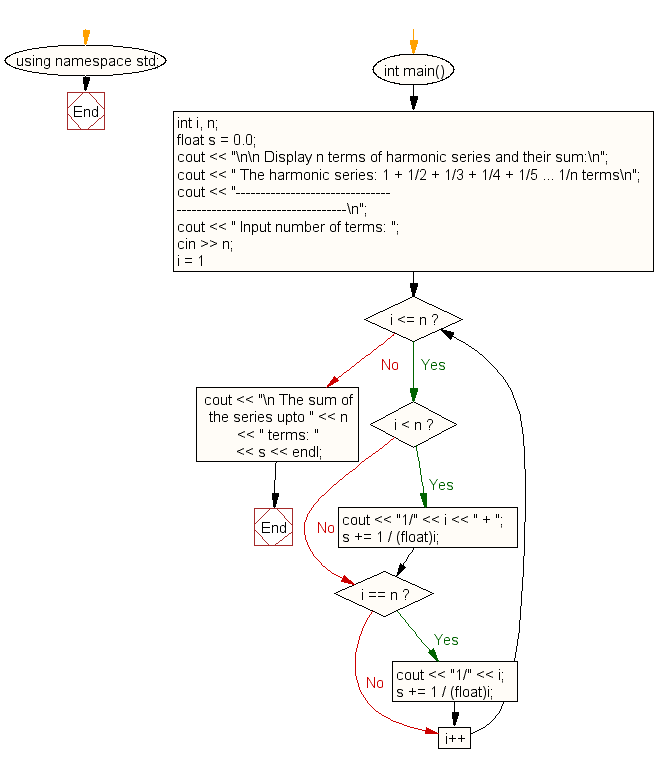
For more Practice: Solve these Related Problems:
- Write a C++ program to print the first n terms of the harmonic series and calculate the sum with floating-point precision.
- Write a C++ program that reads the number of terms and outputs each term of the harmonic series along with the cumulative sum.
- Write a C++ program to compute the harmonic series up to n terms and display the series in a formatted output.
- Write a C++ program that calculates and prints the harmonic series and its total sum using a for loop.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the n terms of even natural number and their sum.
Next: Write a program in C++ to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
What is the difficulty level of this exercise?