C++ Exercises: Display the n terms of odd natural number and their sum
20. Sum of n Odd Natural Numbers
Write a C++ program that displays the sum of n odd natural numbers.
Visual Presentation:
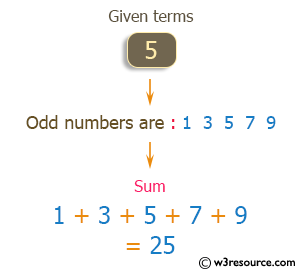
Sample Solution:-
C++ Code :
#include <iostream> // Include input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int i, n, sum = 0; // Declaration of integer variables 'i' (loop counter), 'n' (user input for the number of terms), 'sum' (sum of odd natural numbers)
cout << "\n\n Display n terms of odd natural number and their sum:\n"; // Display message on the console
cout << "---------------------------------------------------------\n";
cout << " Input number of terms: "; // Prompt the user to input the number of terms
cin >> n; // Read the input value as the number of terms
cout << " The odd numbers are: "; // Display message indicating the list of odd numbers
for (i = 1; i <= n; i++) // Loop for 'n' terms
{
cout << 2 * i - 1 << " "; // Print the odd numbers by formula 2 * 'i' - 1
sum += 2 * i - 1; // Add the odd number to the sum variable
}
cout << "\n The Sum of odd Natural Numbers up to " << n << " terms: " << sum << endl; // Display the sum of odd numbers up to 'n' terms
}
Sample Output:
Display n terms of odd natural number and their sum: --------------------------------------------------------- Input number of terms: 5 The odd numbers are: 1 3 5 7 9
Flowchart:
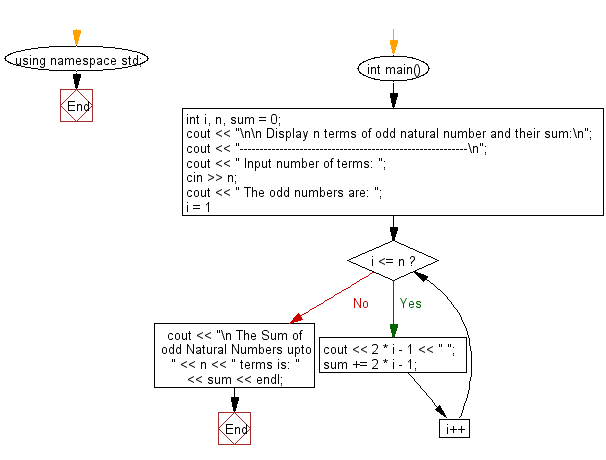
For more Practice: Solve these Related Problems:
- Write a C++ program to list the first n odd natural numbers and compute their sum using a loop.
- Write a C++ program that reads a number n, prints the first n odd numbers, and calculates their total.
- Write a C++ program to generate an array of the first n odd numbers and then sum the elements.
- Write a C++ program that outputs the sequence of n odd numbers and the sum, using a conditional operator to generate odd numbers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the multiplication table vertically from 1 to n.
Next: Write a program in C++ to display the n terms of even natural number and their sum.
What is the difficulty level of this exercise?