C++ Exercises: Asked user to input positive integers to process count, maximum, minimum, and average
15. Process Positive Integers Until Termination Signal (-1)
Write a C++ program that asks the user to enter positive integers in order to process count, maximum, minimum, and average or terminate the process with -1.
Visual Presentation:
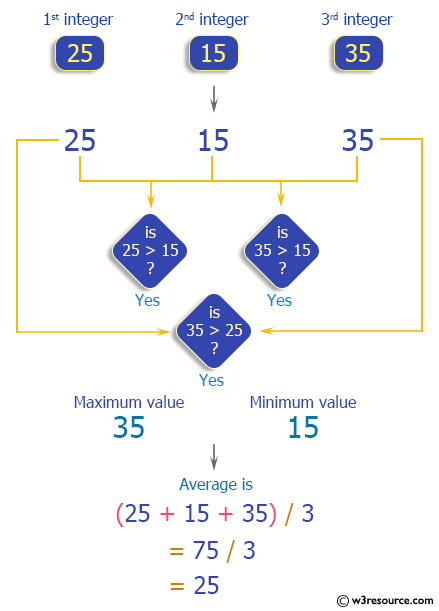
Sample Solution :-
C++ Code :
#include <iostream> // Include input-output stream header
#include <climits> // Include limits header for INT_MAX
#include <iomanip> // Include header for input/output manipulation
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int posnum, ctr = 0, sum = 0; // Declaration of integer variables 'posnum', 'ctr', 'sum' and initialization to 0
int max = 0; // Initialization of 'max' to 0
int min = INT_MAX; // Initialization of 'min' to the maximum integer value available in the system
int terval = -1; // Variable 'terval' initialized with -1 as a termination condition
// Display a message to input positive integers or -1 to terminate
cout << "\n\n Input a positive integers to calculate some processes or -1 to terminate:\n";
cout << "----------------------------------------------------------------------------\n";
cout << " Input positive integer or " << terval << " to terminate: ";
// Read input until termination condition is met (-1 is entered)
while (cin >> posnum && posnum != terval)
{
if (posnum > 0) // Check if the input is positive
{
++ctr; // Increment the counter for positive numbers
sum += posnum; // Calculate sum of positive numbers
if (max < posnum)
max = posnum; // Update maximum value if the current input is larger
if (min > posnum)
min = posnum; // Update minimum value if the current input is smaller
}
else
{
// Display an error message for negative inputs or inputs other than -1
cout << "error: input must be positive! if negative, the value will only be -1! try again..." << endl;
}
// Prompt for the next input or termination
cout << " Input positive integer or " << terval << " to terminate: ";
}
// Display the result after termination
cout << "\n Your input is for termination. Here is the result below: " << endl;
cout << " Number of positive integers is: " << ctr << endl;
if (ctr > 0)
{
cout << " The maximum value is: " << max << endl;
cout << " The minimum value is: " << min << endl;
cout << fixed << setprecision(2); // Set output to fixed precision of 2 decimal places
cout << " The average is " << (double)sum / ctr << endl; // Calculate and display the average of positive integers
}
}
Sample Output:
Input a positive integers to calculate some processes or -1 to terminate: ---------------------------------------------------------------------------- Input positive integer or -1 to terminate: 25 Input positive integer or -1 to terminate: 15 Input positive integer or -1 to terminate: 35 Input positive integer or -1 to terminate: -1 Your input is for termination. Here is the result below: Number of positive integers is: 3 The maximum value is: 35 The minimum value is: 15 The average is 25.00
Flowchart:
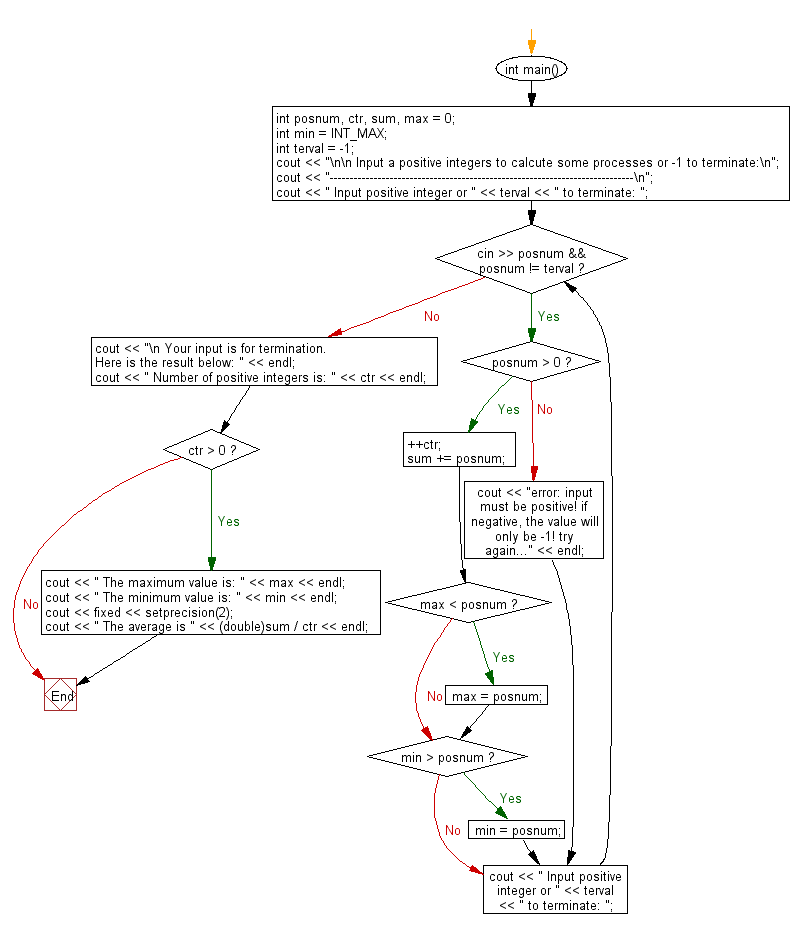
For more Practice: Solve these Related Problems:
- Write a C++ program that continuously accepts positive integers and computes count, maximum, minimum, and average until -1 is entered.
- Write a C++ program to read numbers until -1 is encountered, then display statistics (count, max, min, average) for the positive integers entered.
- Write a C++ program that collects positive integer inputs until a termination value (-1) is received, then outputs summary statistics.
- Write a C++ program that processes user inputs, stops at -1, and calculates the count, maximum, minimum, and average of the positive numbers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of series 1 - X^2/2! + X^4/4!-.... upto nth term.
Next: Write a program in C++ to list non-prime numbers from 1 to an upperbound.
What is the difficulty level of this exercise?