C++ Exercises: Find the sum of series 1 - X^2/2! + X^4/4!-.... upto nth term
14. Sum of Series 1 - X^2/2! + X^4/4! - ... up to nth Term
Write a program in C++ to find the sum of series 1 - X^2/2! + X^4/4!-.... upto nth term.
Sample Solution :-
C++ Code :
#include <iostream> // Including the input/output stream header file
#include <math.h> // Including the math header file for mathematical functions
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
float x, sum, term, fct, y, j, m; // Declaration of float variables 'x', 'sum', 'term', 'fct', 'y', 'j', and 'm'
int i, n; // Declaration of integer variables 'i' and 'n'
y = 2; // Initializing 'y' to 2
// Display a message to find the sum of the series 1 - X^2/2! + X^4/4!-....
cout << "\n\n Find the sum of the series 1 - X^2/2! + X^4/4!-....:\n";
cout << "---------------------------------------------------------\n";
// Prompt the user to input the value of X
cout << " Input the value of X: ";
cin >> x; // Read the value of X entered by the user
// Prompt the user to input the value for nth term
cout << " Input the value for nth term: ";
cin >> n; // Read the value for nth term entered by the user
sum = 1; // Initializing 'sum' to 1
term = 1; // Initializing 'term' to 1
cout << " term 1 value is: " << term << endl; // Display the value of the first term which is always 1
for (i = 1; i < n; i++) // Loop to calculate the series up to the nth term
{
fct = 1; // Initializing 'fct' to 1
for (j = 1; j <= y; j++) // Loop to calculate factorial for the current 'y'
{
fct = fct * j; // Calculating the factorial
}
term = term * (-1); // Changing the sign of the term in the series alternately
m = pow(x, y) / fct; // Calculating the term value using the power function and factorial
m = m * term; // Applying the alternating sign to the term
cout << " term " << i + 1 << " value is: " << m << endl; // Display the value of the current term
sum = sum + m; // Add the current term to the sum
y += 2; // Increment 'y' by 2 for the next iteration (as powers increase by 2 each time)
}
// Display the total sum of the series
cout << " The sum of the above series is: " << sum << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the sum of the series 1 - X^2/2! + X^4/4!-....: --------------------------------------------------------- Input the value of X: 3 Input the value for nth term: 4 term 1 value is: 1 term 2 value is: -4.5 term 3 value is: 3.375 term 4 value is: -1.0125 The sum of the above series is: -1.1375
Flowchart:
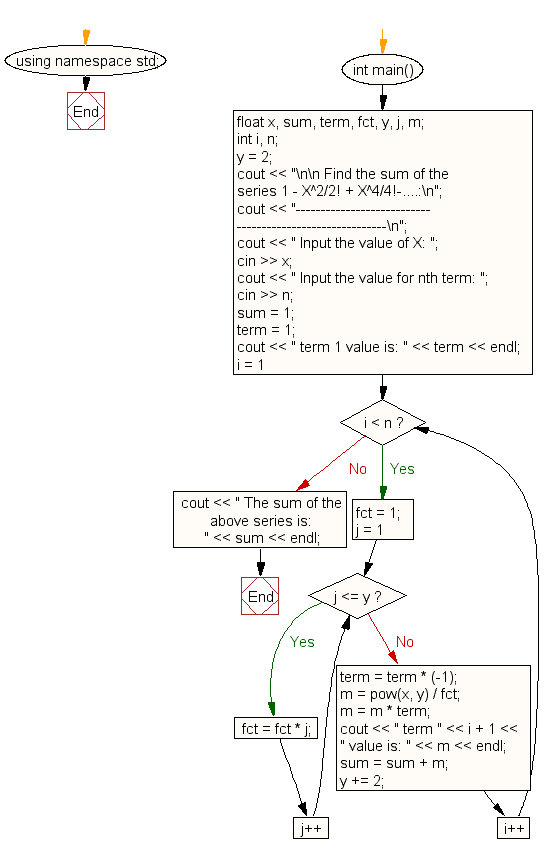
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the alternating series 1 - x^2/2! + x^4/4! - ... for a given x and n using a loop.
- Write a C++ program that reads x and n, then calculates the series sum with alternating signs and factorial denominators.
- Write a C++ program to evaluate the series 1 - x²/2! + x⁴/4! - ... and display each term’s value before summing.
- Write a C++ program that computes the alternating series up to n terms using an iterative approach and prints the final sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to calculate the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + (1+2+3+4+...+n).
Next: Write a program in C++ to asked user to input positive integers to process count, maximum, minimum,
and average or terminate the process with -1.
What is the difficulty level of this exercise?