C++ Exercises: Calculate the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + (1+2+3+4+...+n)
Write a program in C++ to calculate the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + (1+2+3+4+...+n).
Visual Presentation:
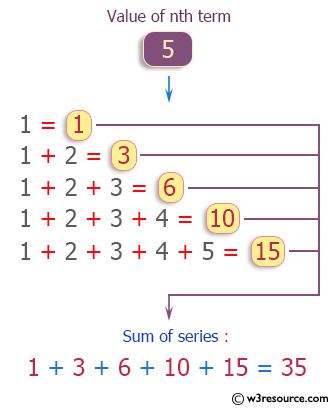
Sample Solution :-
C++ Code :
#include <iostream> // Including the input/output stream header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int i, j, n, sum = 0, tsum; // Declaration of integer variables 'i', 'j', 'n', 'sum', and 'tsum'
// Display a message to find the sum of the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + (1+2+3+4+...+n)
cout << "\n\n Find the sum of the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + (1+2+3+4+...+n):\n";
cout << "------------------------------------------------------------------------------------------\n";
// Prompt the user to input the value for the nth term of the series
cout << " Input the value for nth term: ";
cin >> n; // Read the value entered by the user
for (i = 1; i <= n; i++) // Outer loop to iterate from 1 to 'n'
{
tsum = 0; // Initializing 'tsum' to 0 for each iteration of the outer loop
for (j = 1; j <= i; j++) // Inner loop to calculate the partial sums for each term of the series
{
sum += j; // Add 'j' to 'sum'
tsum += j; // Add 'j' to 'tsum' for the current term
cout << j; // Display the current value of 'j'
if (j < i) // Condition to print '+' for all values of 'j' except the last one in each term
{
cout << "+"; // Display '+'
}
}
cout << " = " << tsum << endl; // Display the calculated partial sum for the current term
}
// Display the total sum of the series
cout << " The sum of the above series is: " << sum << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the sum of the series (1) + (1+2) + (1+2+3) + (1+2+3+4) + ... + ( 1+2+3+4+...+n): ----------------------------------------------------------------------- ------------------- Input the value for nth term: 5 1 = 1 1+2 = 3 1+2+3 = 6 1+2+3+4 = 10 1+2+3+4+5 = 15 The sum of the above series is: 35
Flowchart:
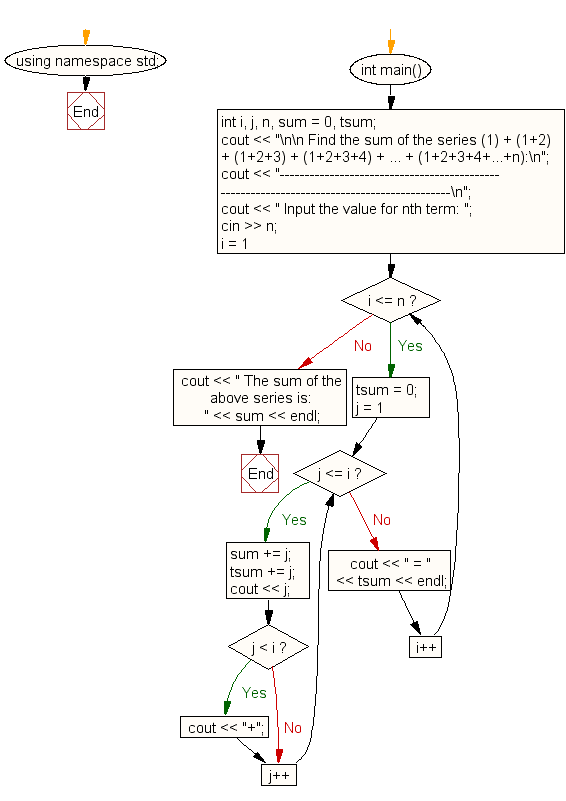
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to calculate the sum of the series (1*1) + (2*2) + (3*3) + (4*4) + (5*5) + ... + (n*n).
Next: Write a program in C++ to find the sum of series 1 - X^2/2! + X^4/4!-.... upto nth term.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics