C++ Exercises: Find the sum of digits of a given number
10. Sum of the Digits of a Given Number
Write a program in C++ to find the sum of the digits of a given number.
Visual Presentation:
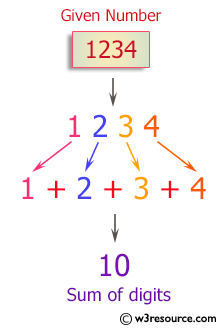
Sample Solution :-
C++ Code :
#include <iostream> // Preprocessor directive to include the input/output stream header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int num1, num2, r, sum = 0; // Declaration of integer variables 'num1', 'num2', 'r', and 'sum'
// Display a message to find the sum of digits of a given number
cout << "\n\n Find the sum of digits of a given number:\n";
cout << "----------------------------------------------\n";
// Prompt the user to input a number
cout << " Input a number: ";
cin >> num1; // Reading the number entered by the user
num2 = num1; // Store the original number in 'num2' for displaying later
// Loop to extract each digit and calculate their sum
while (num1 > 0)
{
r = num1 % 10; // Extract the rightmost digit of 'num1'
num1 = num1 / 10; // Remove the rightmost digit from 'num1'
sum = sum + r; // Add the extracted digit to the 'sum' variable
}
// Display the sum of digits of the original number 'num2'
cout << " The sum of digits of " << num2 << " is: " << sum << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the sum of digits of a given number: ---------------------------------------------- Input a number: 1234 The sum of digits of 1234 is: 10
Flowchart:
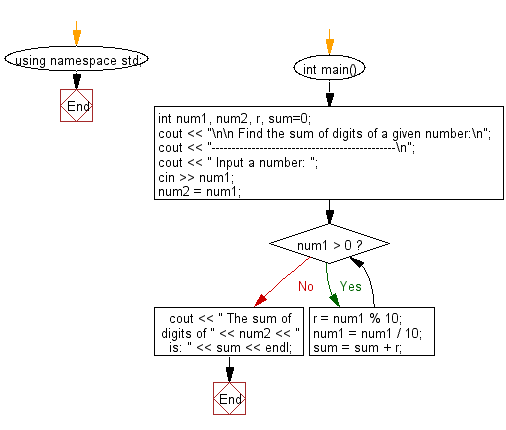
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of digits of an input number using a while loop.
- Write a C++ program that converts an integer to a string and sums its digits by iterating over the characters.
- Write a C++ program to recursively calculate the sum of the digits of a given number.
- Write a C++ program that extracts each digit from a number using modulus and division, and then prints their sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Greatest Common Divisor (GCD) of two numbers.
Next: Write a program in C++ to find the sum of the series 1 + 1/2^2 + 1/3^3 + …..+ 1/n^n.
What is the difficulty level of this exercise?