C++ File handling: Append data to existing text file
7. Append New Data to an Existing Text File
Write a C++ program to append new data to an existing text file.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
int main() {
displayFileContent("new_test.txt"); // Display content of "new_test.txt" before any modification
std::cout << std::endl;
std::ofstream outputFile; // Declare an output file stream object
// Open the file in append mode
outputFile.open("new_test.txt", std::ios::app); // Open "new_test.txt" in append mode
displayFileContent("new_test.txt"); // Display content of "new_test.txt" after opening in append mode
std::cout << std::endl;
if (outputFile.is_open()) { // Check if the file was successfully opened
std::string newData; // Declare a string to store new data entered by the user
std::cout << "Enter the data to append: "; // Prompt the user to enter data
// Read the new data from the user
std::getline(std::cin, newData); // Get user input for new data
// Append the new data to the file
outputFile << newData << std::endl; // Write the new data to the file
outputFile.close(); // Close the file
std::cout << "Data appended successfully." << std::endl; // Display a success message
displayFileContent("new_test.txt"); // Display content of "new_test.txt" after appending data
std::cout << std::endl;
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
File content: CPP is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern CPP currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide CPP compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. File content: CPP is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern CPP currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide CPP compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. Enter the data to append: Here are some exercies of C++. Data appended successfully. File content: CPP is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern CPP currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide CPP compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. Here are some exercies of C++.
Explanation:
In the above exercise -
- Use the std::ofstream class to open the file in append mode.
- The std::ios::app flag is passed as the second argument to the open() function, which ensures that new data is appended to the existing file content.
- Check if the file was successfully opened using the is_open() function.
- If the file is open, prompt the user to input the data they want to append to the file. The std::getline() function reads new data from the user.
- Next use the output stream operator << to append the new data to the file.
- The std::endl inserts a new line character after the new data.
- After appending the data, close the file using outputFile.close(), and display a message.
Note: Content of a file is displayed using displayFileContent("filename.txt").
Flowchart:
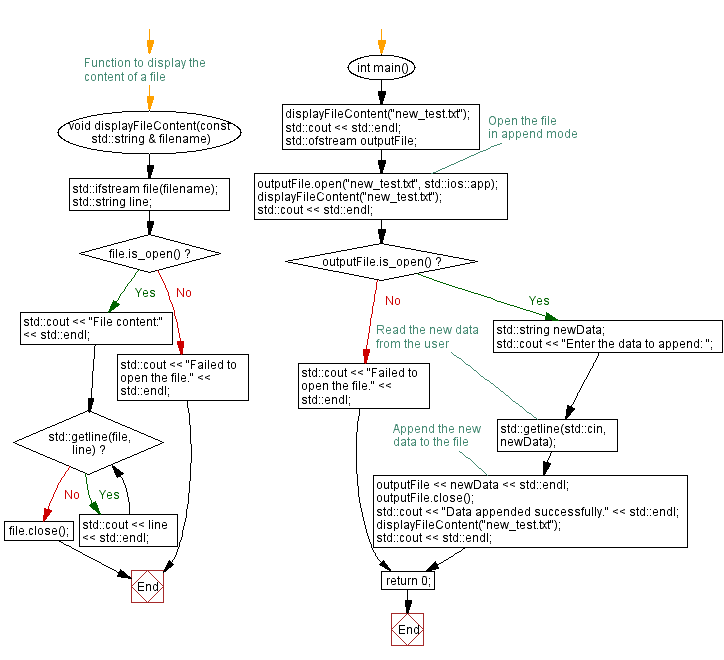
For more Practice: Solve these Related Problems:
- Write a C++ program to open a text file in append mode and add a new line of text at the end.
- Write a C++ program that appends user-inputted data to an existing file, preserving its original content.
- Write a C++ program to dynamically append multiple lines to a text file and then display the updated content.
- Write a C++ program that opens a file in append mode, writes additional data, and verifies that the data was appended by reopening the file.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find and replace specific word in text file.
Next C++ Exercise: Sort text file lines alphabetically.
What is the difficulty level of this exercise?