C++ File handling: Count number of words in a text file
Write a C++ program to count the number of words in a text file.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
#include <sstream> // Including the stringstream library
int main() {
std::ifstream inputFile("test.txt"); // Open the text file named "test.txt" for reading
if (inputFile.is_open()) { // Checking if the file was successfully opened
std::string line; // Declaring a string variable to store each line of text
int wordCount = 0; // Initializing a variable to count words
while (std::getline(inputFile, line)) { // Loop through each line in the file
std::stringstream ss(line); // Create a stringstream object with the current line content
std::string word; // Declare a string variable to store each word
while (ss >> word) { // Extract words from the stringstream
wordCount++; // Increment word count for each word extracted
}
}
inputFile.close(); // Closing the file after counting words
std::cout << "Number of words in the said file: " << wordCount << std::endl; // Outputting the total word count
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Number of words in the said file: 76
Explanation:
In the above exercise,
- Use the std::ifstream class to open the text file.
- Check if the file was successfully opened using the is_open() function.
- If the file is open, declare an int variable wordCount to keep track of the number of words.
- Then use a while loop and std::getline() to read each line from the file. Inside this loop, use std::stringstream to break the line into individual words.
- Use another while loop with ss >> word to extract each word and increment the wordCount for each word encountered.
- Display the total number of words on the console after the loops end using inputFile.close().
Flowchart:
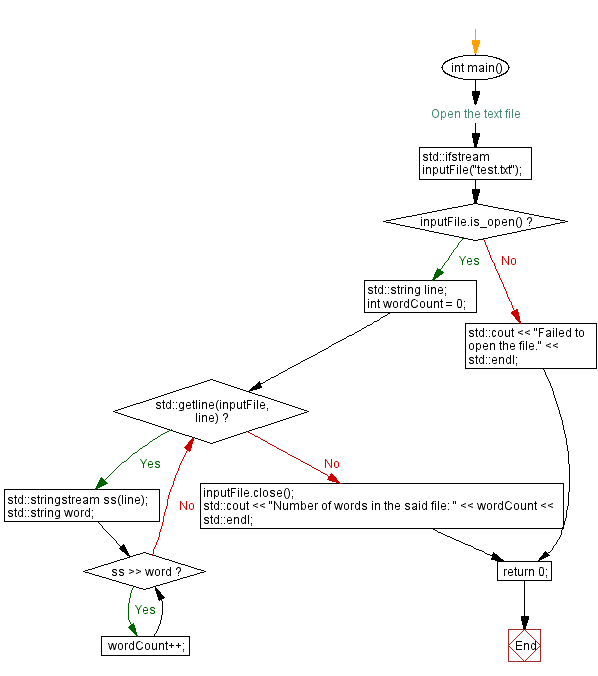
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Open and display text file contents.
Next C++ Exercise: Copy contents from one text file to another.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics