C++ File handling: Count number of lines in a text file
Write a C++ program to count the number of lines in a text file.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
int main() {
// Open the text file
std::ifstream inputFile("test.txt"); // Opening the file named "test.txt" for reading
if (inputFile.is_open()) { // Checking if the file was successfully opened
std::string line; // Declaring a string variable to store each line of text
int lineCount = 0; // Initializing a variable to count lines
while (std::getline(inputFile, line)) { // Loop through each line in the file
lineCount++; // Incrementing line count for each line read
}
inputFile.close(); // Closing the file after counting lines
std::cout << "Number of lines in the file: " << lineCount << std::endl; // Outputting the total line count
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Number of lines in the file: 5
Explanation:
In the above exercise -
- Use the std::ifstream class to open the text file.
- Check if the file was successfully opened using the is_open() function.
- If the file is open, declare an int variable lineCount to keep track of the number of lines.
- Use a while loop and std::getline() to read each line from the file and increment the lineCount for each line read.
- After the loop ends, close the file using inputFile.close() and display the total number of lines on the console.
Flowchart:
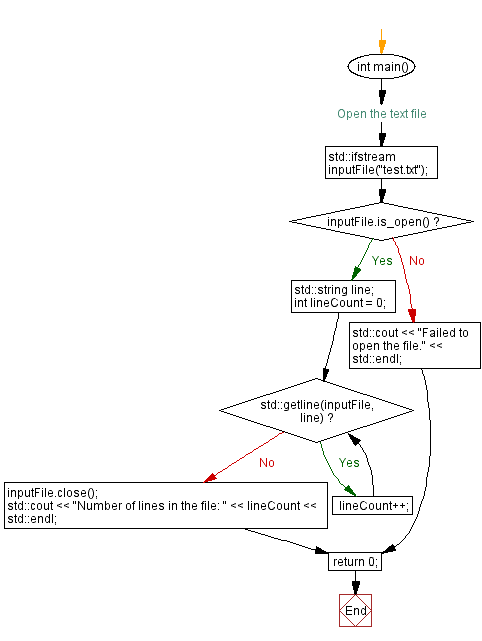
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Open and display text file contents.
Next C++ Exercise: Count number of words in a text file.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics