C++ File handling: Open and display text file contents
2. Open and Display File Contents
Write a C++ program to open an existing text file and display its contents on the console.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
int main() {
// Open an existing file named "test.txt"
std::ifstream inputFile("test.txt"); // Opening the file named "test.txt" for reading
if (inputFile.is_open()) { // Checking if the file was successfully opened
std::string line; // Declaring a string variable to store each line of text
while (std::getline(inputFile, line)) { // Loop through each line in the file
// Display each line on the console
std::cout << line << std::endl; // Output the content of 'line' to the console
}
inputFile.close(); // Closing the file after reading
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM.
Explanation:
In the above exercise -
- Use the std::ifstream class to open an existing file named "example.txt".
- Check if the file was successfully opened using the is_open() function.
- If the file is open, use a while loop and std::getline() to read each line from the file and store it in the line variable.
- Display each line on the console using std::cout.
After running this program, it will open the "example.txt" file (assuming it exists in the same directory as the program) and display its contents on the console.
Flowchart:
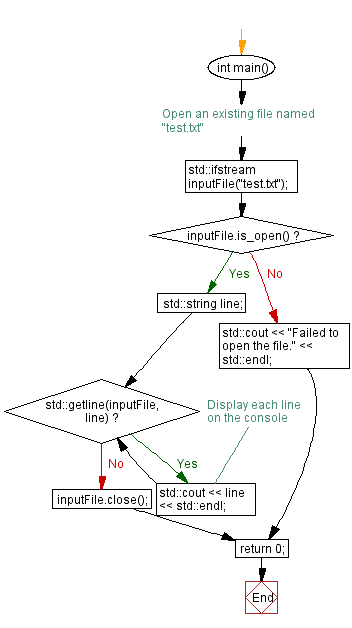
For more Practice: Solve these Related Problems:
- Write a C++ program to open an existing text file and print its contents line by line on the console.
- Write a C++ program that reads a text file into a string and then displays the complete text on the screen.
- Write a C++ program to open a file in input mode and display its content using a while loop until EOF is reached.
- Write a C++ program that uses ifstream to read and output the content of a file, handling errors if the file cannot be opened.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Create text file and write text.
Next C++ Exercise: Count number of lines in a text file.
What is the difficulty level of this exercise?