C++ File handling: Encrypt text file using a simple algorithm
12. Encrypt Text File Using a Simple Algorithm
Write a C++ program to encrypt the contents of a text file using a simple encryption algorithm.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
// Function to encrypt a file using a simple algorithm (incrementing ASCII values)
void encryptFile(const std::string & inputFile, const std::string & outputFile) {
std::ifstream input(inputFile); // Open input file for reading
std::ofstream output(outputFile); // Open or create output file for writing
if (input.is_open() && output.is_open()) { // Check if both files were successfully opened
char ch; // Declare a character variable to read characters from the input file
while (input.get(ch)) { // Loop through each character in the input file
ch++; // Simple encryption algorithm: Increment ASCII value by 1
output.put(ch); // Write the encrypted character to the output file
}
input.close(); // Close the input file
output.close(); // Close the output file
std::cout << "File encrypted successfully.\n" << std::endl; // Display a success message
} else {
std::cout << "Failed to open the files.\n" << std::endl; // Display an error message if file opening failed
}
}
int main() {
std::string inputFile = "test.txt"; // Input file
displayFileContent("test.txt"); // Display content of "test.txt"
std::cout << std::endl; // Output a newline for formatting
std::string outputFile = "encrypted_test.txt"; // Output file for encrypted content
encryptFile(inputFile, outputFile); // Encrypt "test.txt" and write to "encrypted_test.txt"
displayFileContent("encrypted_test.txt"); // Display content of "encrypted_test.txt"
std::cout << std::endl; // Output a newline for formatting
return 0; // Return 0 to indicate successful execution
}
Sample Output:
File content: C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. File encrypted successfully. File content: D,,!jt!b!ijhi.mfwfm-!hfofsbm.qvsqptf!qsphsbnnjoh!mbohvbhf!dsfbufe!cz!Ebojti!dpnqvufs!tdjfoujtu!Ckbsof!Tuspvtusvq/!♂Gjstu!sfmfbtfe!jo!2:96!bt!bo!fyufotjpo!pg!uif!D!qsphsbnnjoh!mbohvbhf-!ju!ibt!tjodf!fyqboefe!tjhojgjdboumz!pwfs!ujnf/!♂Npefso!D,,!dvssfoumz!ibt!pckfdu.psjfoufe-!hfofsjd-!boe!gvodujpobm!gfbuvsft-!jo!beejujpo!up!gbdjmjujft!gps!mpx.mfwfm!nfnpsz!nbojqvmbujpo/♂Ju!jt!bmnptu!bmxbzt!jnqmfnfoufe!jo!b!dpnqjmfe!mbohvbhf/♂Nboz!wfoepst!qspwjef!D,,!dpnqjmfst-!jodmvejoh!uif!Gsff!Tpguxbsf!Gpvoebujpo-!MMWN-!Njdsptpgu-!Joufm-!Fncbsdbefsp-!Psbdmf-!boe!JCN/
Explanation:
In the above exercise,
- The function encryptFile() takes two parameters: inputFile (the name of the input file to encrypt) and outputFile (the name of the output file to store the encrypted content).
- The program opens the input file using std::ifstream and the output file using std::ofstream.
- It then reads each character from the input file using std::ifstream::get and applies a simple encryption algorithm by incrementing the ASCII value of each character by 1.
- The encrypted character is written to the output file using std::ofstream::put.
- After encrypting the entire file, both the input and output files are closed, and a success message is displayed.
Note: Content of a file is displayed using displayFileContent("filename.txt").
Flowchart:
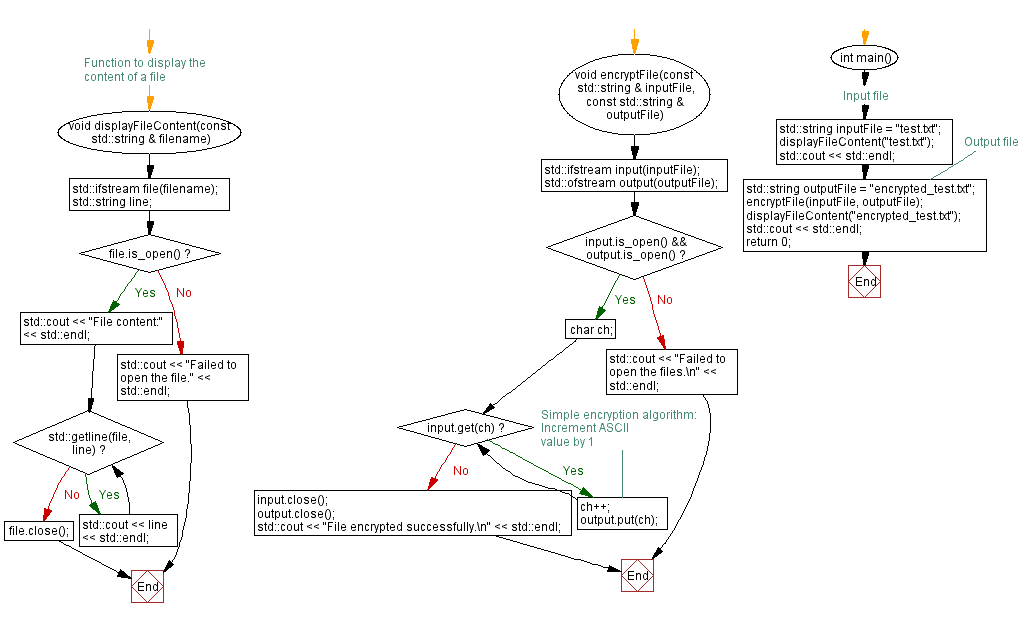
For more Practice: Solve these Related Problems:
- Write a C++ program to encrypt a text file using a simple Caesar cipher and save the encrypted content to a new file.
- Write a C++ program that reads a text file, applies a basic shift encryption to each character, and writes the result to an output file.
- Write a C++ program to implement a simple substitution cipher to encrypt the contents of a text file.
- Write a C++ program that encrypts a file by shifting each character by a fixed number and then prints the encrypted message.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Search for string with line number in text file.
Next C++ Exercise: Decrypting the contents of a text file.
What is the difficulty level of this exercise?