C++ File handling: Search for string with line number in text file
11. Search for a Specific String in a Text File and Display Line Numbers
Write a C++ program to search for a specific string in a text file and display its line number(s).
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
#include <vector> // Including the vector container
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
// Function to search for a string in a file and display line numbers where it is found
void searchAndDisplayLineNumbers(const std::string & filename, const std::string & searchStr) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
std::vector<int> lineNumbers; // Vector to store line numbers where the search string is found
int lineNumber = 1; // Variable to track the current line number
while (std::getline(file, line)) { // Read each line from the file
if (line.find(searchStr) != std::string::npos) { // Check if the search string is found in the line
lineNumbers.push_back(lineNumber); // Store the line number where the search string is found
}
lineNumber++; // Increment the line number counter
}
file.close(); // Close the file after reading
if (!lineNumbers.empty()) { // Check if any line numbers were stored
std::cout << "String \"" << searchStr << "\" found at line number(s): "; // Display a message indicating the search string was found
for (int i = 0; i < lineNumbers.size(); ++i) { // Loop through the stored line numbers
std::cout << lineNumbers[i]; // Display each line number
if (i != lineNumbers.size() - 1) {
std::cout << ", "; // Display a comma between line numbers, except for the last one
}
}
std::cout << std::endl; // Move to the next line after displaying line numbers
} else {
std::cout << "String \"" << searchStr << "\" not found in the file." << std::endl; // Display a message indicating the search string was not found
}
}
int main() {
std::string filename = "test.txt"; // File to search
displayFileContent("new_test.txt"); // Display content of "new_test.txt"
std::cout << std::endl; // Output a newline for formatting
std::string searchStr = "currently"; // String to search
searchAndDisplayLineNumbers(filename, searchStr); // Search for the string in the file and display line numbers where it's found
return 0; // Return 0 to indicate successful execution
}
Sample Output:
File content: CPP is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Modern CPP currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. It is almost always implemented in a compiled language. Many vendors provide CPP compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. Here are some exercies of C++. String "currently" found at line number(s): 3
Explanation:
In the above exercise -
- The function searchAndDisplayLineNumbers() takes two parameters: filename (the name of the text file to search) and searchStr (the string to search for).
- The program opens the file using std::ifstream and iterates over each line using std::getline.
- For each line, it checks if it contains the search string using std::string::find. If the search string is found in a line, it stores the line number in a std::vector<int>.
- After searching the entire file, the input file is closed.
- If the lineNumbers vector is not empty, it displays the line number(s) where the search string was found.
- Otherwise, it displays a message indicating that the search string was not found.
Note: Content of a file is displayed using displayFileContent("filename.txt").
Flowchart:
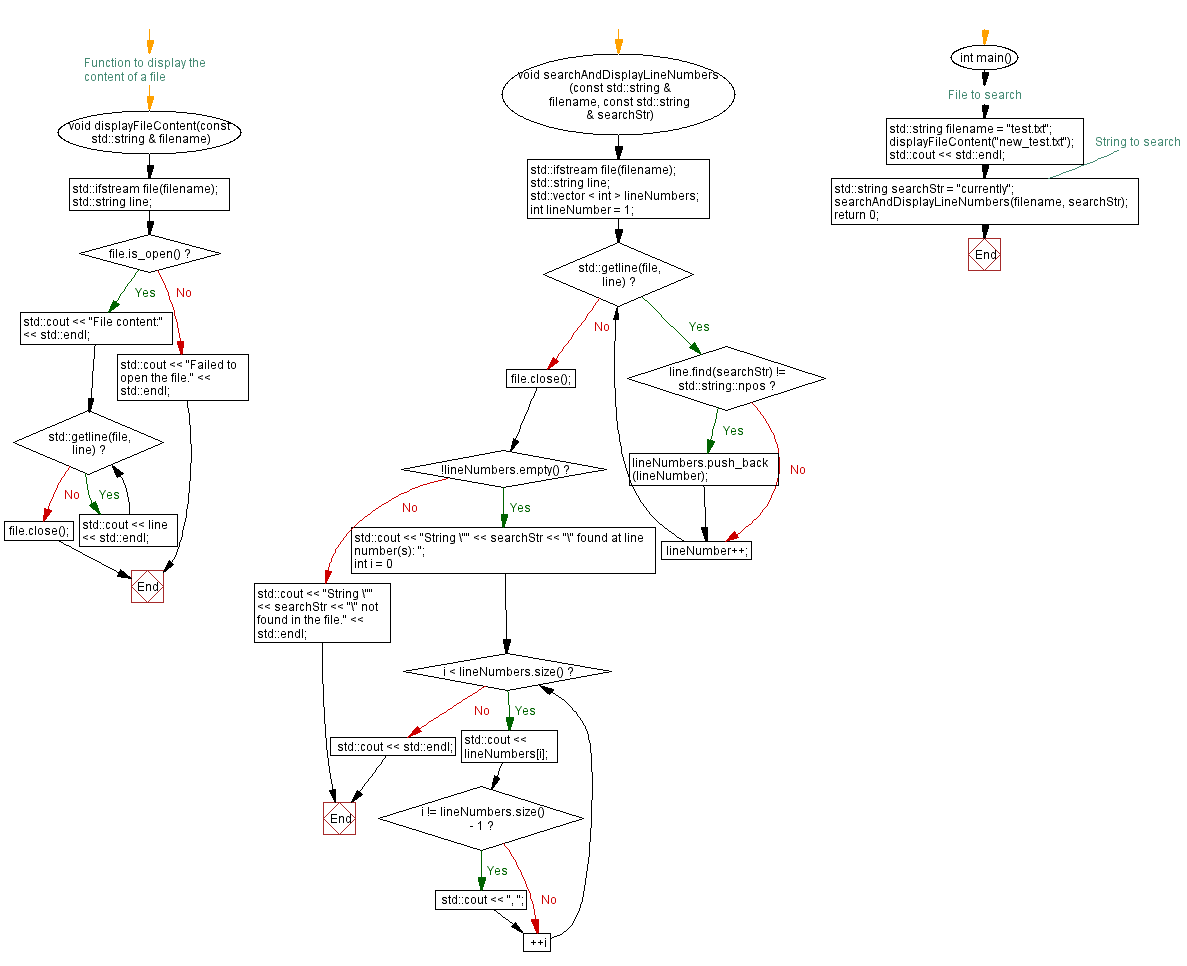
For more Practice: Solve these Related Problems:
- Write a C++ program to search a text file for a given substring and print out all line numbers where it occurs.
- Write a C++ program that reads a file and outputs the line numbers and text lines containing a user-specified string.
- Write a C++ program to scan a file for a target word and display the lines and corresponding line numbers where it is found.
- Write a C++ program that opens a text file, searches for a string, and highlights the line numbers where the string appears.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Split a large text file.
Next C++ Exercise: Encrypt text file using a simple algorithm.
What is the difficulty level of this exercise?