C++ File handling: Create text file and write text
1. Create and Write to a Text File
Write a C++ program to create a new text file and write some text into it.
Sample Solution:
C Code:
#include <iostream> // Include the input/output stream library
#include <fstream> // Include the file stream library
int main() {
// Create a new file named "test.txt"
std::ofstream outputFile("test.txt"); // Open/create a file named "test.txt" for writing
if (outputFile.is_open()) { // Check if the file was successfully opened
// Write some text into the file
outputFile << "C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. \n"; // Write a line of text to the file
outputFile << "First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. \n"; // Write a line of text to the file
outputFile << "Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation.\n"; // Write a line of text to the file
outputFile << "It is almost always implemented in a compiled language.\n"; // Write a line of text to the file
outputFile << "Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM."; // Write a line of text to the file
// Close the file
outputFile.close(); // Close the file after writing
std::cout << "Text has been written to the file." << std::endl; // Display a success message
} else {
std::cout << "Failed to create the file." << std::endl; // Display an error message if file creation failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Text has been written to the file.
Explanation:
In the above exercise -
- Use the std::ofstream class to create a new file named "test.txt".
- Check if the file was successfully opened using the is_open() function.
- If the file is open, use the output stream operator << to write text to the file.
- Finally, close the file using the close() function.
After running this program, a new file named "example.txt" will be created in the same directory as the program, and the specified text will be written into it.
Flowchart:
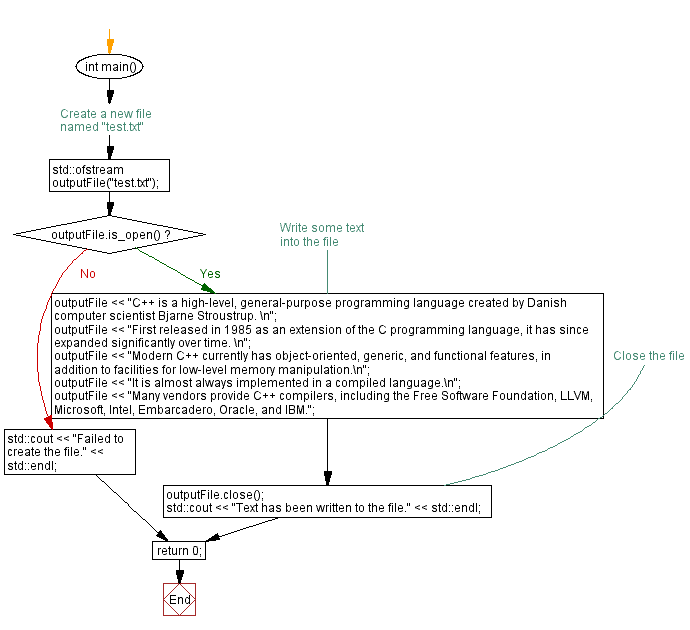
For more Practice: Solve these Related Problems:
- Write a C++ program to dynamically create a text file and write multiple lines of text into it using file streams.
- Write a C++ program that opens a file in output mode, writes user input into the file, and then closes the file.
- Write a C++ program to create a text file, write a formatted message to it, and then display a confirmation message.
- Write a C++ program that accepts text from the user and saves it into a new file, ensuring proper error handling for file operations.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: C++ File handling Exercises Home.
Next C++ Exercise: Open and display text file contents.
What is the difficulty level of this exercise?