C++ Dynamic Memory Allocation: Stack implementation with memory allocation
9. Dynamically Allocate Memory for a Stack and Implement Push/Pop
Write a C++ program to dynamically allocate memory for a stack data structure. Implement push and pop operations on this stack.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
class Stack {
private:
int * array; // Dynamic array to store the stack elements
int top; // Index of the top element
int capacity; // Maximum capacity of the stack
public:
// Constructor to initialize the stack with a given size
Stack(int size) {
capacity = size;
array = new int[capacity]; // Allocate memory for the stack
top = -1; // Initialize top as -1 to indicate an empty stack
}
// Destructor to deallocate memory when the object is destroyed
~Stack() {
delete[] array; // Free the dynamically allocated memory for the stack
}
// Function to push an element onto the stack
void push(int value) {
if (top == capacity - 1) { // Check for stack overflow
std::cout << "Stack Overflow. Cannot push element: " << value << std::endl;
return;
}
array[++top] = value; // Increment top and add the element to the stack
std::cout << "Pushed element: " << value << std::endl; // Print the pushed element
}
// Function to pop an element from the stack
void pop() {
if (top == -1) { // Check for stack underflow
std::cout << "Stack Underflow. Cannot pop from an empty stack." << std::endl;
return;
}
int value = array[top--]; // Get the top element and decrement top
std::cout << "Popped element: " << value << std::endl; // Print the popped element
}
};
int main() {
int size = 5; // Size of the stack
std::cout << "Size of the stack: " << size << "\n" << std::endl; // Print the stack size
Stack stack(size); // Create an instance of the Stack class with the specified size
// Push elements onto the stack
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
// Pop elements from the stack
std::cout << "\nPopped 6 elements from the above stack:" << std::endl;
stack.pop();
stack.pop();
stack.pop();
stack.pop();
stack.pop();
stack.pop(); // Attempting to pop from an empty stack
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Size of the stack: 5 Pushed element: 1 Pushed element: 2 Pushed element: 3 Pushed element: 4 Pushed element: 5 Popped 6 elements from the above stack: Popped element: 5 Popped element: 4 Popped element: 3 Popped element: 2 Popped element: 1 Stack Underflow. Cannot pop from an empty stack.
Explanation:
In the above exercise,
push(): This function adds elements to the stack. It checks if the stack is already full (top == capacity - 1). If it is, it displays an error message indicating a stack overflow. Otherwise, it increments the top index and assigns the provided value to the corresponding position in the array.
pop(): This function removes elements from the stack. It checks if the stack is empty (top == -1). If it is, it displays an error message indicating a stack underflow. Otherwise, it retrieves the value from the top position in the array, decrements the top index, and displays the popped element.
Flowchart:
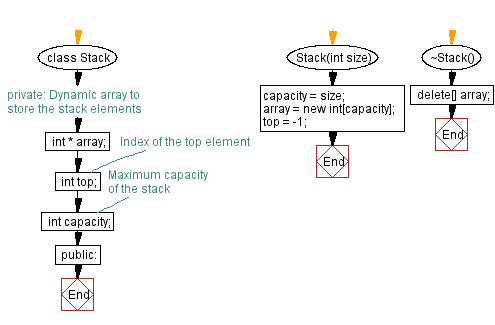
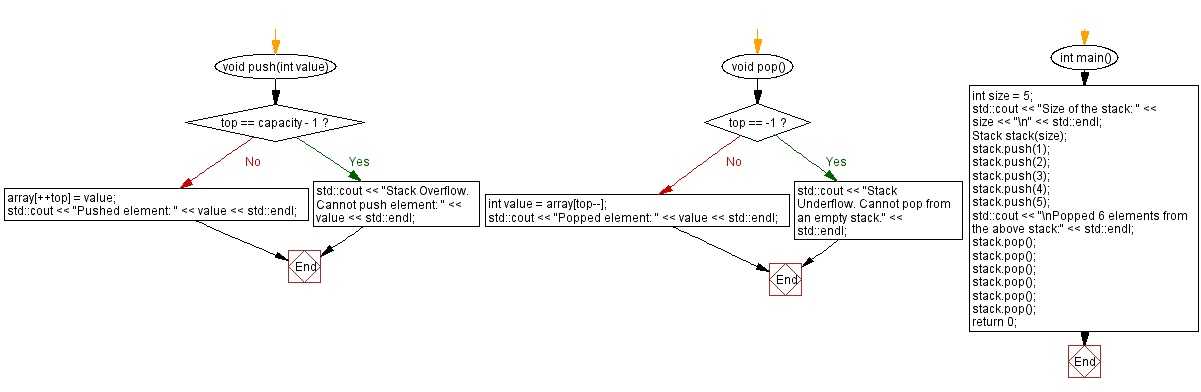
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a stack using dynamic memory allocation, supporting push and pop operations with error handling.
- Write a C++ program that dynamically allocates a stack of integers, then simulates a series of push and pop operations and prints the stack content.
- Write a C++ program to create a dynamic stack data structure using linked list implementation, and then perform basic operations such as push, pop, and display.
- Write a C++ program that uses the new operator to dynamically allocate memory for a stack and implements methods for pushing, popping, and checking if the stack is empty.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Linked list operations with memory allocation.
Next C++ Exercise: Queue implementation with memory allocation .
What is the difficulty level of this exercise?