C++ Dynamic Memory Allocation: Allocating memory for structure and user input
C++ Dynamic Memory Allocation: Exercise-7 with Solution
Write a C++ program to dynamically allocate memory for a structure and input its members from the user.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
// Defining a structure named MyStruct
struct MyStruct {
int id; // Integer member variable 'id'
std::string name; // String member variable 'name'
std::string country; // String member variable 'country'
};
int main() {
// Dynamically allocate memory for a structure of type MyStruct
MyStruct * EmployeeStruct = new MyStruct; // Allocating memory for a single instance of MyStruct
// Input structure members from the user
std::cout << "Employee ID (int value): ";
std::cin >> EmployeeStruct->id; // Reading user input into the 'id' member of EmployeeStruct
std::cout << "Input Employee name: ";
std::cin.ignore(); // Ignoring the newline character from the previous input
std::getline(std::cin, EmployeeStruct->name); // Reading user input into the 'name' member of EmployeeStruct
std::cout << "Input Country name: ";
std::getline(std::cin, EmployeeStruct->country); // Reading user input into the 'country' member of EmployeeStruct
// Display the structure members
std::cout << "\nEmployee Details:" << std::endl;
std::cout << "ID: " << EmployeeStruct->id << std::endl; // Outputting the 'id' member of EmployeeStruct
std::cout << "Name: " << EmployeeStruct->name << std::endl; // Outputting the 'name' member of EmployeeStruct
std::cout << "Country: " << EmployeeStruct->country << std::endl; // Outputting the 'country' member of EmployeeStruct
// Deallocate the dynamically allocated structure
delete EmployeeStruct; // Deallocating the memory occupied by the EmployeeStruct pointer
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Employee ID (int value): 12 Input Employee name: Teuna Vinicio Input Country name: France Employee Details: ID: 12 Name: Teuna Vinicio Country: France
Explanation:
In the above exercise,
- We define a structure called MyStruct with two members: id of type int and name of type std::string.
- Inside the main() function, we use the new operator to dynamically allocate memory for a MyStruct structure. We assign its address to a pointer called dynamicStruct. This dynamically allocated structure resides in heap memory.
- Next prompt the user to input the structure members: id, name and country using std::cin.
- We then display the input structure members using std::cout and the arrow operator (->) to access the dynamic structure members.
- Finally, we deallocate the dynamically allocated structure using the delete operator to free the memory occupied by the structure.
Flowchart:
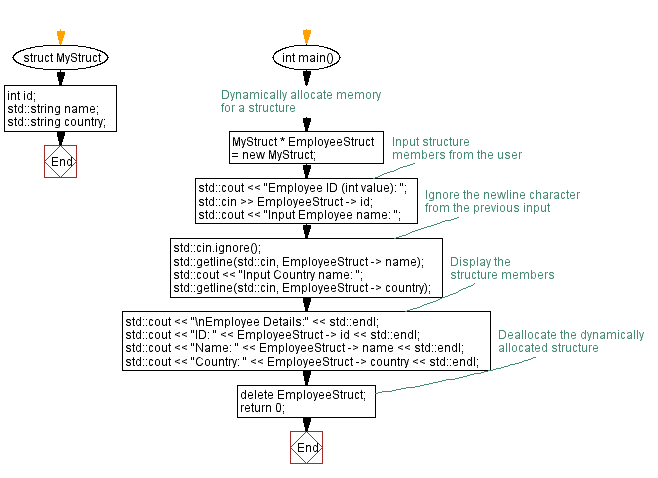
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Creating an array of objects with new operator.
Next C++ Exercise: Linked list operations with memory allocation.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/dynamic-memory-allocation/cpp-dynamic-memory-allocation-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics