C++ Dynamic Memory Allocation: Creating an array of objects with new operator
6. Dynamically Create an Array of Objects Using New
Write a C++ program to dynamically create an array of objects using the new operator.
Sample Solution:
C Code:
Sample Output:
Input the size of the array: 10 Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object! Dynamic object!
Explanation:
In the above exercise,
- We define a class called MyClass with a member function displayMessage() that outputs a message.
- Inside the main() function, prompt the user to input the array size using std::cin. We store the size in the size variable.
- We then use the new operator to dynamically create an array of MyClass objects and assign its address to a pointer called dynamicArray. This dynamically created array resides in the heap memory.
- Next we use a for loop to access and use each object in the dynamic array. In this example, we call the displayMessage() member function of each object.
- Finally, we deallocate the dynamically created array using the delete[] operator to free the memory occupied by the array.
Flowchart:
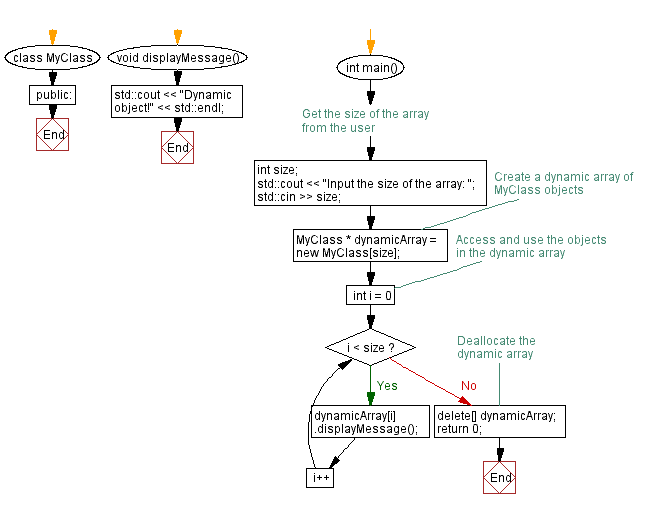
For more Practice: Solve these Related Problems:
- Write a C++ program to define a class for a book and then dynamically allocate an array of book objects, initializing and printing their details.
- Write a C++ program that creates a dynamic array of objects of a class representing a point in 2D space and prints their coordinates.
- Write a C++ program to dynamically allocate an array of objects of a class (e.g., Employee) and input values for each object from the user.
- Write a C++ program that uses new to create an array of objects for a class with a parameterized constructor, then displays the array contents.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Creating Objects with new Operator.
Next C++ Exercise: Allocating memory for structure and user input.
What is the difficulty level of this exercise?