C++ Dynamic Memory Allocation: Input character and string
Write a C++ program to dynamically allocate memory for a character and a string. Input a character and a string from the user.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
#include <string> // Including the String Library
int main() {
// Dynamically allocate memory for a character
char * dynamicChar = new char; // Allocating memory to store a single character
// Input a character from the user
std::cout << "Input a character: ";
std::cin >> * dynamicChar; // Taking a single character input and storing it in the dynamically allocated memory
// Dynamically allocate memory for a string
std::string * dynamicString = new std::string; // Allocating memory to store a string
// Input a string from the user
std::cout << "Input a string: ";
std::cin.ignore(); // Ignoring the newline character from the previous input
std::getline(std::cin, * dynamicString); // Taking a string input and storing it in the dynamically allocated memory for string
// Display the input character and string
std::cout << "Input character: " << * dynamicChar << std::endl; // Outputting the input character
std::cout << "Input string: " << * dynamicString << std::endl; // Outputting the input string
// Deallocate the dynamically allocated memory
delete dynamicChar; // Deallocating the memory allocated for the character
delete dynamicString; // Deallocating the memory allocated for the string
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Input a character: C Input a string: C++ Exercises Input character: C Input string: C++ Exercises
Explanation:
In the above exercise,
- First use the new operator to dynamically allocate memory for a character and a string. The character is allocated with new char and the string is allocated with new std::string.
- Next prompt the user to input a character using std::cin >> *dynamicChar. The >> operator extracts the character from the user input and store it in the dynamically allocated character.
- Again prompt the user to input a string using std::getline(std::cin, *dynamicString). The std::getline function reads the entire text line, including any spaces, and stores it in the dynamically allocated string.
- Finally, display the input character and string using std::cout.
Flowchart:
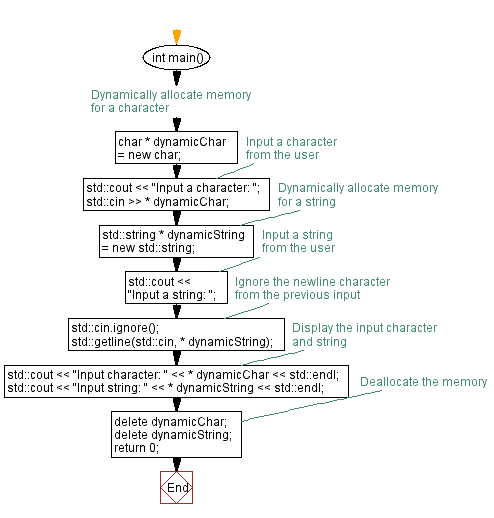
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Initializing 2D arrays of floats and strings.
Next C++ Exercise: Creating Objects with new Operator.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics