C++ Dynamic Memory Allocation: Array of integers and strings initialization
C++ Dynamic Memory Allocation: Exercise-2 with Solution
Write a C++ program to dynamically allocate an array of integers and strings and initialize its elements.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
#include <string> // Including the String Library
int main() {
// Dynamically allocate an array of integers
int size = 7; // Size of the array
int * dynamicIntArray = new int[size]; // Allocating memory for an integer array of 'size' elements
// Initialize the elements of the integer array
for (int i = 0; i < size; i++) {
dynamicIntArray[i] = i + 1; // Setting values in the dynamically allocated integer array
}
// Dynamically allocate an array of strings
std::string * dynamicStringArray = new std::string[size]; // Allocating memory for a string array of 'size' elements
// Initialize the elements of the string array
for (int i = 0; i < size; i++) {
dynamicStringArray[i] = "Element-" + std::to_string(i + 1); // Setting values in the dynamically allocated string array
}
// Display the elements of the dynamically allocated integer array
std::cout << "Dynamically allocated integer array:" << std::endl;
for (int i = 0; i < size; i++) {
std::cout << dynamicIntArray[i] << " "; // Outputting each element of the dynamically allocated integer array
}
std::cout << std::endl;
// Display the elements of the dynamically allocated string array
std::cout << "\nDynamically allocated string array:" << std::endl;
for (int i = 0; i < size; i++) {
std::cout << dynamicStringArray[i] << std::endl; // Outputting each element of the dynamically allocated string array
}
// Deallocate the memory allocated for both arrays
delete[] dynamicIntArray; // Deallocating memory for the dynamically allocated integer array
delete[] dynamicStringArray; // Deallocating memory for the dynamically allocated string array
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Dynamically allocated integer array: 1 2 3 4 5 6 7 Dynamically allocated string array: Element-1 Element-2 Element-3 Element-4 Element-5 Element-6 Element-7
Explanation:
In the above exercise -
- Use the new operator to dynamically allocate memory for an array of integers and strings. The array size is determined by the variable size.
- Initialize the integer array with values from 1 to size using a for loop. Similarly, initialize the string array elements with strings like "Element 1", "Element 2", etc.
- Next display the elements of both arrays using std::cout. The integer array elements are displayed on a single line separated by spaces, while the string array elements are displayed one per line.
- Finally, deallocate dynamically allocated memory using the delete[] operator for both arrays.
Flowchart:
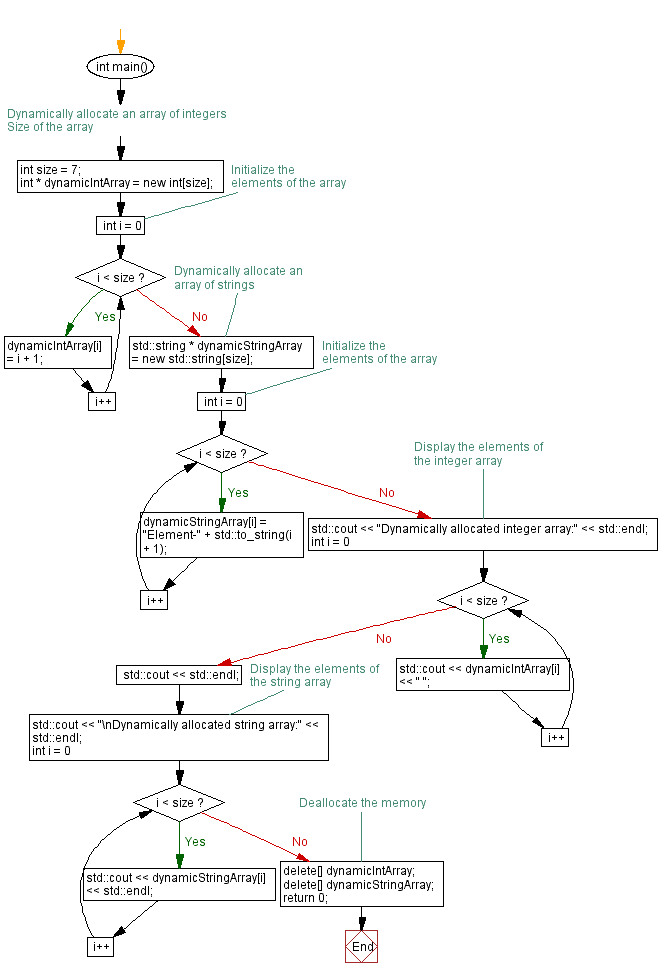
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Integer, character, string assignment.
Next C++ Exercise: Initializing 2D arrays of floats and strings.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics