C++ Dynamic Memory Allocation: Queue implementation with memory allocation
10. Dynamically Allocate Memory for a Queue and Implement Enqueue/Dequeue
Write a C++ program to dynamically allocate memory for a queue data structure. Implement enqueue and dequeue operations on this queue.
Sample Solution:
C Code:
#include <iostream> // Including the Input/Output Stream Library
class Queue {
private:
int * nums; // Dynamic array to store the queue elements
int front; // Index of the front element
int rear; // Index of the rear element
int capacity; // Maximum capacity of the queue
int size; // Current size of the queue
public:
// Constructor to initialize the queue with a given size
Queue(int queueSize) {
capacity = queueSize;
nums = new int[capacity]; // Allocate memory for the queue
front = rear = -1; // Initialize front and rear as -1 to indicate an empty queue
size = 0; // Initialize size as 0
}
// Destructor to deallocate memory when the object is destroyed
~Queue() {
delete[] nums; // Free the dynamically allocated memory for the queue
}
// Function to enqueue an element into the queue
void enqueue(int value) {
if (size == capacity) { // Check for queue overflow
std::cout << "Queue Overflow. Cannot enqueue element: " << value << std::endl;
return;
}
rear = (rear + 1) % capacity; // Update rear index using circular queue logic
nums[rear] = value; // Add the element to the queue
size++; // Increment the size of the queue
if (front == -1) {
front = rear; // If the queue was empty, update the front index
}
std::cout << "Enqueued element: " << value << std::endl; // Print the enqueued element
}
// Function to dequeue an element from the queue
void dequeue() {
if (size == 0) { // Check for queue underflow
std::cout << "Queue Underflow. Cannot dequeue from an empty queue." << std::endl;
return;
}
int value = nums[front]; // Get the front element
front = (front + 1) % capacity; // Update front index using circular queue logic
size--; // Decrement the size of the queue
std::cout << "Dequeued element: " << value << std::endl; // Print the dequeued element
if (size == 0) {
front = rear = -1; // If the queue becomes empty, reset front and rear indices
}
}
};
int main() {
int queueSize = 5; // Size of the queue
std::cout << "Size of the queue: " << queueSize << std::endl; // Print the queue size
Queue queue(queueSize); // Create an instance of the Queue class with the specified size
// Enqueue elements into the queue
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
queue.enqueue(4);
queue.enqueue(5);
// Dequeue elements from the queue
queue.dequeue();
queue.dequeue();
queue.dequeue();
queue.dequeue();
queue.dequeue();
queue.dequeue(); // Attempting to dequeue from an empty queue
return 0; // Returning 0 to indicate successful execution of the program
}
Sample Output:
Size of the queue: 5 Enqueued element: 1 Enqueued element: 2 Enqueued element: 3 Enqueued element: 4 Enqueued element: 5 Dequeued element:1 Dequeued element:2 Dequeued element:3 Dequeued element:4 Dequeued element:5 Queue Underflow. Cannot dequeue from an empty queue
Explanation:
In the above exercise,
The enqueue() function checks if the queue is full (size == capacity) and displays an error message if it is. Otherwise, it increments the rear index circularly wrapping around the array. It assigns the provided value to the rear position of the array, updates the size, and adjusts the front index if necessary.
The dequeue() function checks if the queue is empty (size == 0) and displays an error message if it is. Otherwise, it retrieves the value from the front position in the array. It increments the front index circularly, decrements the size, and adjusts the front and rear indices if necessary.
Flowchart:
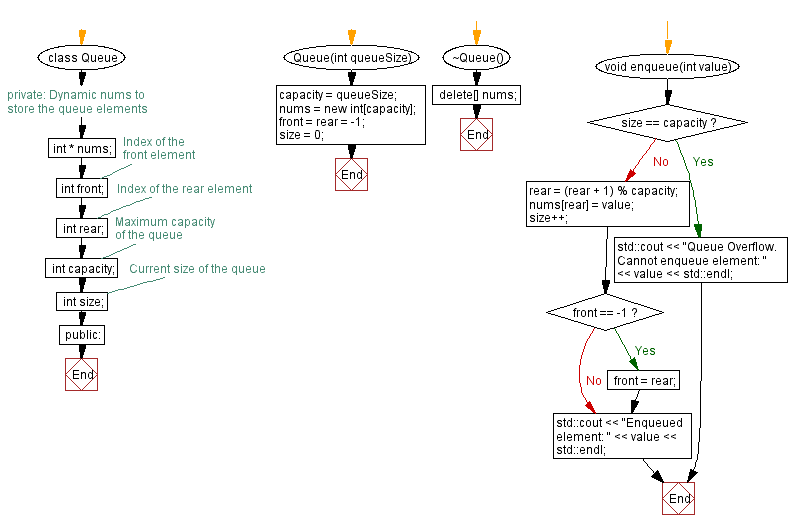
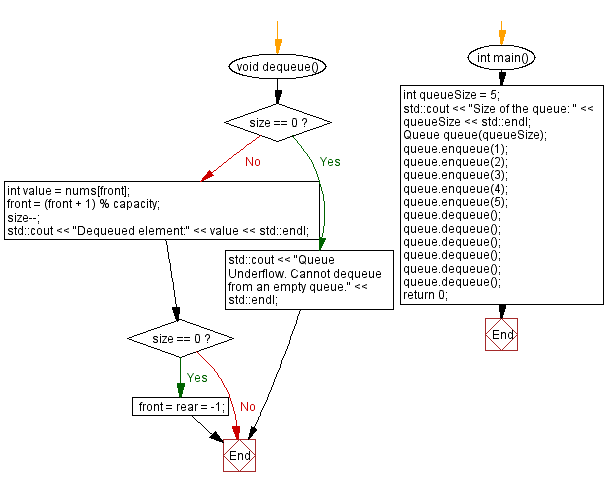
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a queue using dynamic memory allocation and pointers, including functions for enqueue and dequeue.
- Write a C++ program that dynamically allocates memory for a queue of integers using a circular array and implements enqueue and dequeue operations.
- Write a C++ program to create a dynamic queue data structure using linked list implementation, with functions to add and remove elements.
- Write a C++ program that reads input values into a dynamically allocated queue and then processes them using dequeue to display the order of removal.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Stack implementation with memory allocation.
What is the difficulty level of this exercise?