C++ Exercises: Number of days of a month from a given year and month
5. Compute the Number of Days in a Month for a Given Year and Month
Write a C++ program to compute the number of days in a month for a given year and month.
Sample Solution-1:
C++ Code:
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if a year is a leap year or not
bool is_leap_year(int y) {
return y % 400 == 0 || (y % 4 == 0 && y % 100 != 0); // Returns true if the year is divisible by 400 or divisible by 4 but not by 100
}
// Function to get the number of days in a month for a given year
int no_of_days(int month, int year) {
switch (month) { // Switch statement to check the month
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31; // Months with 31 days
break;
case 4:
case 6:
case 9:
case 11:
return 30; // Months with 30 days
break;
case 2:
if (is_leap_year(year) == 1)
return 29; // February in a leap year
else
return 28; // February in a non-leap year
break;
default:
break;
}
return 0; // Return 0 if month input is invalid
}
// Main function
int main() {
int year = 0; // Declaring an integer variable to store the year
int month = 0; // Declaring an integer variable to store the month
int days; // Declaring an integer variable to store the number of days
cout << "Input Year: "; // Output message for user input of year
cin >> year; // Inputting the year value
cout << "Input Month: "; // Output message for user input of month
cin >> month; // Inputting the month value
if (year > 0 && (month > 0 && month < 13)) { // Checking if the year and month inputs are valid
// Displaying the number of days in the given year and month
cout << "Number of days of the year " << year << " and month " << month << " is: " << no_of_days(month, year) << endl;
} else {
cout << "Wrong year/month!"; // Displaying an error message for invalid year/month input
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Input Year: 2019 Input Month: 04 Number of days of the year 2019 and month 4 is: 30
Flowchart:
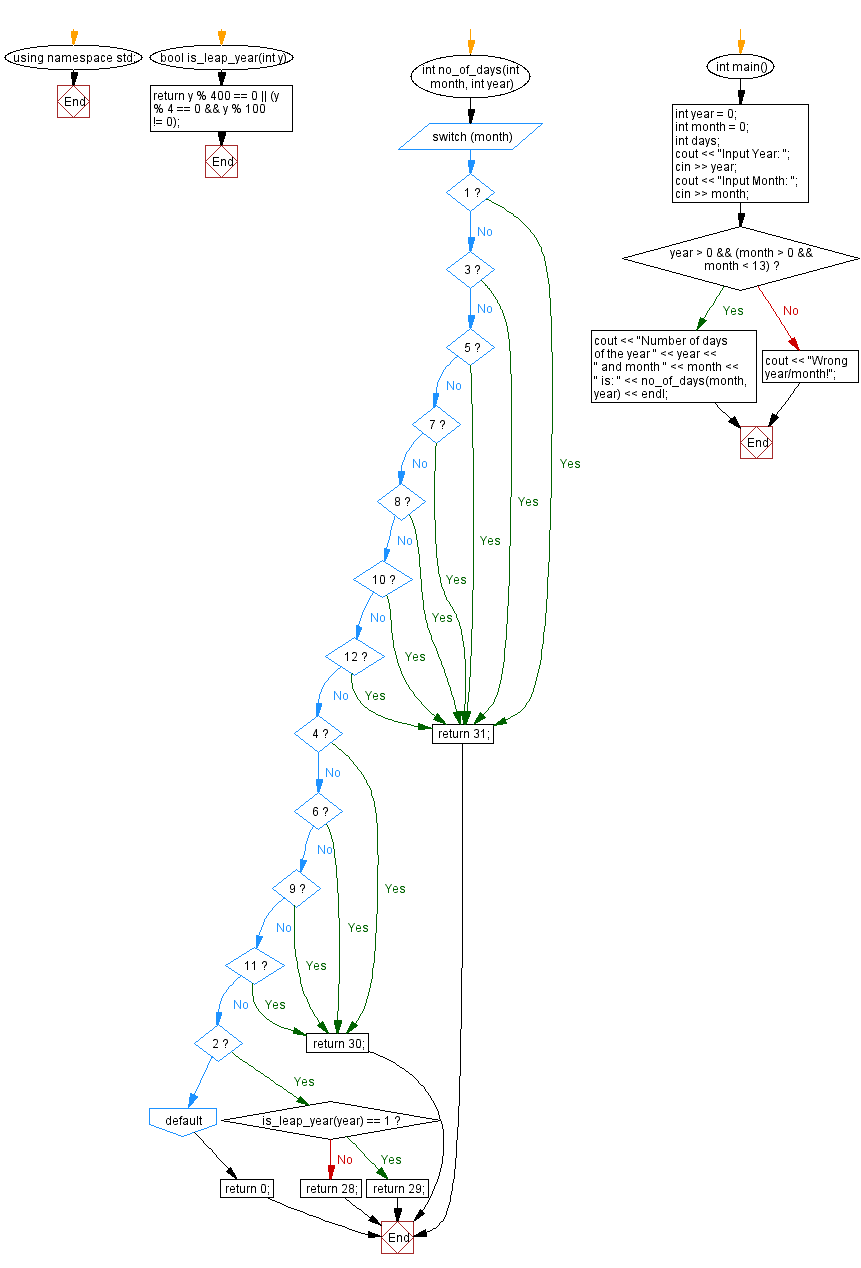
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the number of days in any given month, accounting for leap years in February.
- Write a C++ program that reads a year and a month and uses a switch-case structure to output the correct number of days.
- Write a C++ program to calculate the number of days in a month by storing month-day mappings in an array and adjusting for leap years.
- Write a C++ program that validates user input for month and year, then outputs the number of days in that month, handling invalid inputs appropriately.
Sample Solution-2:
C++ Code:
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to get the number of days in a month for a given year
int no_of_days(int month, int year) {
if (month == 4 || month == 6 || month == 9 || month == 11) // Months with 30 days
{
return 30;
}
else if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) // Months with 31 days
{
return 31;
}
else if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year % 100 == 0 && year % 400 == 0))) // February in a leap year or a non-leap year
{
return 29; // February has 29 days in a leap year
}
else // Default case for February in a non-leap year
{
return 28; // February has 28 days in a non-leap year
}
}
// Main function
int main() {
int year = 0; // Declaring an integer variable to store the year
int month = 0; // Declaring an integer variable to store the month
cout << "Input Year: "; // Output message for user input of year
cin >> year; // Inputting the year value
cout << "Input Month: "; // Output message for user input of month
cin >> month; // Inputting the month value
if (year > 0 && (month > 0 && month < 13)) { // Checking if the year and month inputs are valid
// Displaying the number of days in the given year and month
cout << "Number of days of the year " << year << " and month " << month << " is: " << no_of_days(month, year) << endl;
} else {
cout << "Wrong year/month!"; // Displaying an error message for invalid year/month input
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Input Year: 2019 Input Month: 04 Number of days of the year 2019 and month 4 is: 30
Flowchart:
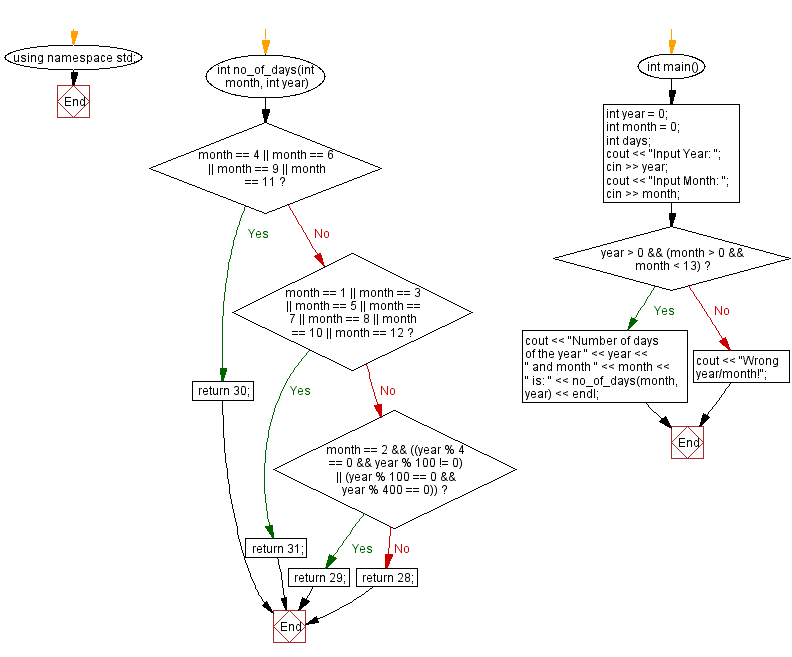
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Check whether a given year is a leap year not.
Next: Count the number of days between two given dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.