C++ Exercises: Check whether a given year is a leap year not
4. Check Whether a Given Year is a Leap Year
Write a C++ program to check whether a given year is a leap year or not.
Sample Solution-1:
C++ Code:
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if a year is a leap year or not
bool is_leap_year(int year) {
if (year % 400 == 0) // If the year is divisible by 400
return true; // It's a leap year
if (year % 100 == 0) // If the year is divisible by 100 but not by 400
return false; // It's not a leap year
if (year % 4 == 0) // If the year is divisible by 4 but not by 100
return true; // It's a leap year
return false; // If none of the above conditions match, it's not a leap year
}
// Main function
int main() {
int y; // Declaring an integer variable to store the year
y = 1900; // Assigning a year value
cout << "Is Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 1999; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2000; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2010; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2020; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Is Leap year: 1900? Century of the year: 0 Is Leap year: 1999? Century of the year: 0 Is Leap year: 2000? Century of the year: 1 Is Leap year: 2010? Century of the year: 0 Is Leap year: 2020? Century of the year: 1
Flowchart:
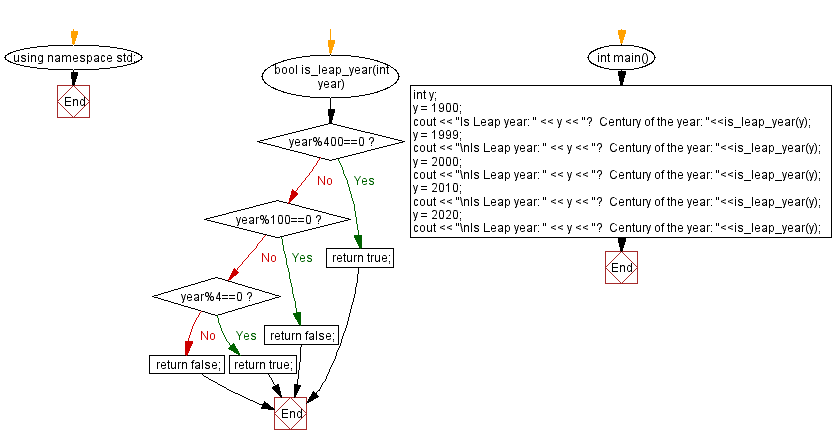
Sample Solution-2:
C++ Code:
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if a year is a leap year or not
bool is_leap_year(int y) {
return y % 400 == 0 || (y % 4 == 0 && y % 100 != 0); // Returns true if the year is divisible by 400 or divisible by 4 but not by 100
}
// Main function
int main() {
int y; // Declaring an integer variable to store the year
y = 1900; // Assigning a year value
cout << "Is Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 1999; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2000; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2010; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
y = 2020; // Assigning a year value
cout << "\nIs Leap year: " << y << "? Century of the year: " << is_leap_year(y); // Display the year and check if it's a leap year
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Is Leap year: 1900? Century of the year: 0 Is Leap year: 1999? Century of the year: 0 Is Leap year: 2000? Century of the year: 1 Is Leap year: 2010? Century of the year: 0 Is Leap year: 2020? Century of the year: 1
Flowchart:
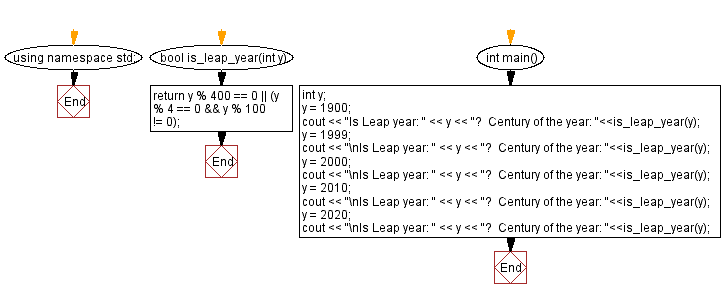
For more Practice: Solve these Related Problems:
- Write a C++ program that determines if a year is a leap year using the standard rules and outputs "Leap Year" or "Not a Leap Year."
- Write a C++ program to check if a given year is a leap year by incorporating a function that validates century years correctly.
- Write a C++ program that reads a list of years and prints which ones are leap years, using a loop and conditional statements.
- Write a C++ program to check for leap years and also compute the number of days in February for the given year, outputting the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Convert a given year to century.
Next: Number of days of a month from a given year and month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.