C++ Exercises: Convert a given year to century
3. Convert a Given Year to a Century
Write a C++ program to convert a given year to a century.
Note: A century is a period of 100 years. Centuries are numbered ordinally in English and many other languages. The word century comes from the Latin centum, meaning one hundred. Century is sometimes abbreviated as c.
Sample Solution-1:
C++ Code:
#include <iostream>
#include <cmath> // Including the cmath library for mathematical functions
using namespace std; // Using the standard namespace
// Function to determine the century of a given year
double year_to_century(int y) {
return floor((y - 1) / 100 + 1); // Calculate the century by dividing the year by 100 and rounding down to the nearest integer
}
// Main function
int main() {
int y; // Declaring an integer variable to store the year
y = 1900; // Assigning a year value
cout << "Year: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 1999; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2000; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2010; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2020; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Year: 1900, Century of the year: 19 Year: 1999, Century of the year: 20 Year: 2000, Century of the year: 20 Year: 2010, Century of the year: 21 Year: 2020, Century of the year: 21
Flowchart:
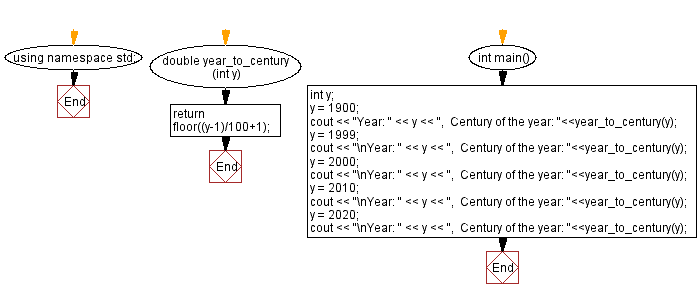
Sample Solution-2:
C++ Code:
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to determine the century of a given year
double year_to_century(int y) {
return (y - 1) / 100 + 1; // Calculating the century by dividing the year by 100 and adding 1
}
// Main function
int main() {
int y; // Declaring an integer variable to store the year
y = 1900; // Assigning a year value
cout << "Year: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 1999; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2000; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2010; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
y = 2020; // Assigning a year value
cout << "\nYear: " << y << ", Century of the year: " << year_to_century(y); // Display the year and its corresponding century
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Year: 1900, Century of the year: 19 Year: 1999, Century of the year: 20 Year: 2000, Century of the year: 20 Year: 2010, Century of the year: 21 Year: 2020, Century of the year: 21
Flowchart:
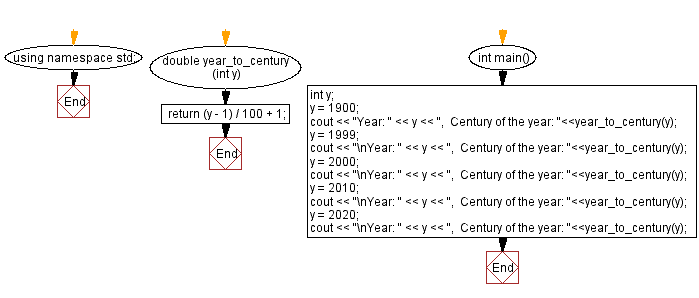
For more Practice: Solve these Related Problems:
- Write a C++ program that converts a given year to its corresponding century, ensuring proper handling of edge cases such as years ending in 00.
- Write a C++ program to read a year and calculate the century using integer division and modulus operators, then output the result with a suffix (e.g., "th").
- Write a C++ program that converts multiple years to centuries by reading from an array of years and displaying their corresponding centuries.
- Write a C++ program to convert a year to a century and then compare it with the current century, outputting whether the year is in the past, current, or future century.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Get the day of the week from a given date.
Next: Check whether a given year is a leap year not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.