C++ Exercises: Get the day of the week from a given date
C++ Date: Exercise-2 with Solution
Write a C++ program to get the day of the week from a given date. Return the day as a string. The format of the day is MM/DD/YYYY.
- YYYY denotes the 4 digit year.
- MM denotes the 2 digit month.
- DD denotes the 2 digit day.
Note: Assume the week starts on Monday.
Sample Solution:
C++ Code:
#include <iostream>
#include <string>
#include <vector> // Including the vector library for using vectors
using namespace std; // Using the standard namespace
// Function to find the day of the week for a given date
std::string day_of_the_week(std::string day) {
int month = stoi(day.substr(0, 2)); // Extracting the month from the date string and converting it to an integer
int _day = stoi(day.substr(3, 2)); // Extracting the day from the date string and converting it to an integer
int year = stoi(day.substr(6, 4)); // Extracting the year from the date string and converting it to an integer
// Vector storing the number of days in each month
vector<int> days = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// Vector storing the names of the days in a week
vector<string> dates = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"};
int d = _day; // Initialize the total days with the given day of the month
// Calculate the total number of days considering the months before the given month
for (int i = 0; i < month - 1; i++)
d += days[i];
// Adjust for leap years, adding an extra day for February if the year is a leap year and the month is after February
if (((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) && month > 2)
d++;
// Calculate the total days considering the years before the given year
for (int i = 1971; i < year; i++) {
if ((i % 4 == 0 && i % 100 != 0) || i % 400 == 0)
d += 366; // Leap year
else
d += 365; // Non-leap year
}
// Calculate the day of the week using the obtained total days, adjusting with a fixed offset
string result = dates[(d % 7 + 3) % 7];
return result; // Return the day of the week
}
// Main function
int main() {
string date1, date2, date3, date4; // Declare strings to store different dates
date1 = "02/05/1980"; // Assign a date
cout << "Date: " << date1 << " Day of the week: " << day_of_the_week(date1); // Display the date and its corresponding day of the week
date2 = "01/24/1990"; // Assign a date
cout << "\nDate: " << date2 << " Day of the week: " << day_of_the_week(date2); // Display the date and its corresponding day of the week
date3 = "01/05/2019"; // Assign a date
cout << "\nDate: " << date3 << " Day of the week: " << day_of_the_week(date3); // Display the date and its corresponding day of the week
date4 = "11/17/2022"; // Assign a date
cout << "\nDate: " << date4 << " Day of the week: " << day_of_the_week(date4); // Display the date and its corresponding day of the week
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Date: 02/05/1980 Day of the week: Tuesday Date: 01/24/1990 Day of the week: Wednesday Date: 01/05/2019 Day of the week: Saturday Date: 11/17/2022 Day of the week: Thursday
N.B.: The result may vary for your current system date and time.
Flowchart:
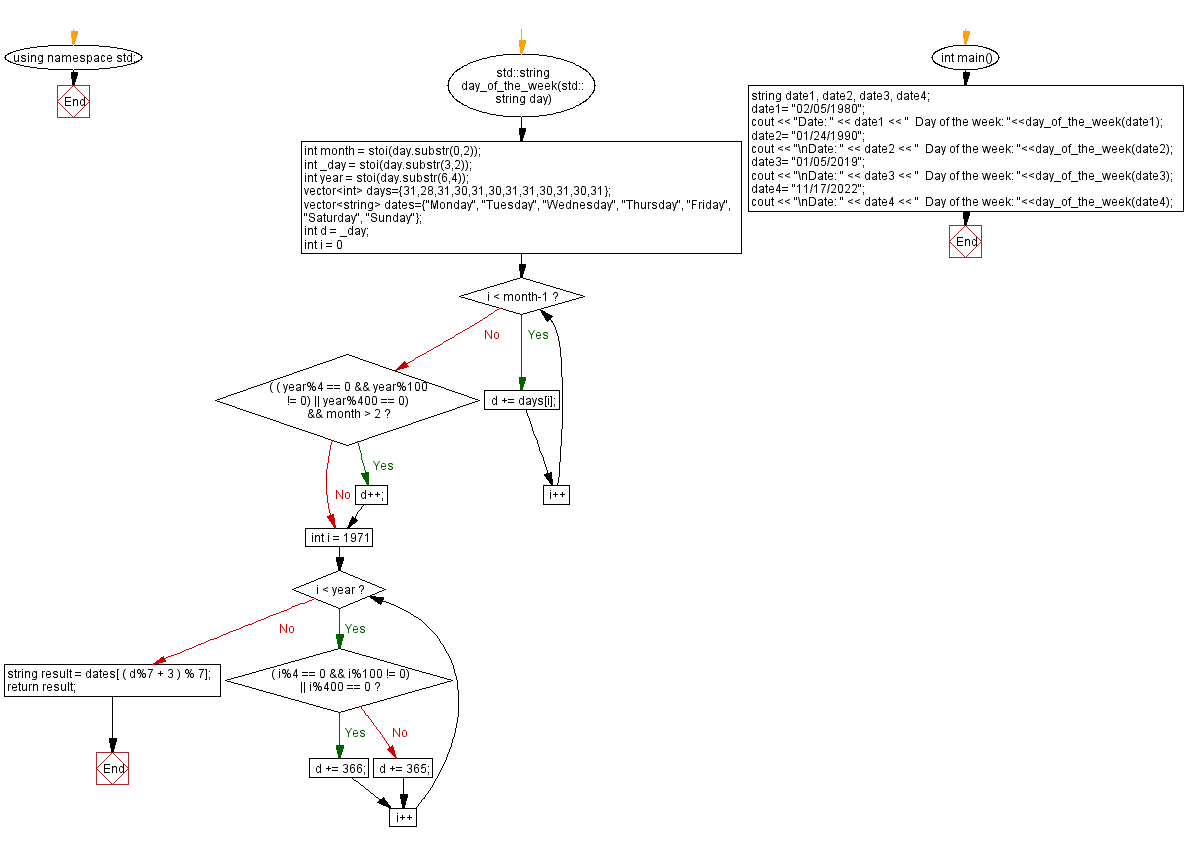
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Current date and time.
Next: Convert a given year to century.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/date/cpp-date-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics