C++ Exercises: Current date and time
C++ Date: Exercise-1 with Solution
Write a C++ program to get the current date and time.
Sample Solution-1:
C++ Code:
#include <iostream>
#include <ctime> // Including the C Standard Library header for time functions
using namespace std;
int main()
{
// current date and time in current system using time_t data type
time_t current_dt = time(0); // Get the current system time in seconds since epoch (Jan 1, 1970)
// convert date time to string format using ctime function
char* result = ctime(¤t_dt); // Convert the time_t value to a string representing local time
// Display the current date and time in string format
cout << "The current date and time is:\n" << result << endl;
}
Sample Output:
The current date and time is: Tue Mar 15 14:28:05 2022
N.B.: The result may vary for your current system date and time.
Flowchart:
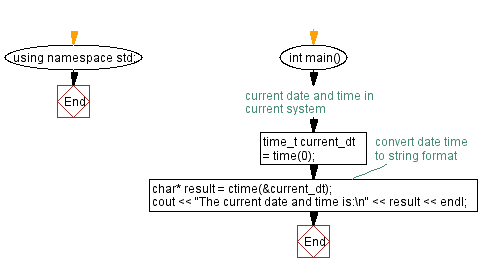
Sample Solution-2:
C++ Code:
#include <iostream>
#include <ctime>
using namespace std;
int main() {
time_t crtime; // Declare a variable to hold the current time as seconds since epoch
struct tm* time_info; // Declare a pointer to a structure to hold time information
char result[80]; // Declare an array to store formatted time as a string
time(&crtime); // Get the current system time in seconds since epoch and store it in crtime
time_info = localtime(&crtime); // Convert the time_t value into a local time representation using localtime function
// Format the time_info into a string according to the specified format "%d-%m-%Y %H:%M:%S"
strftime(result, sizeof(result), "%d-%m-%Y %H:%M:%S", time_info);
cout << result; // Output the formatted time string
return 0;
}
Sample Output:
15-03-2022 12:15:31
N.B.: The result may vary for your current system date and time.
Flowchart:
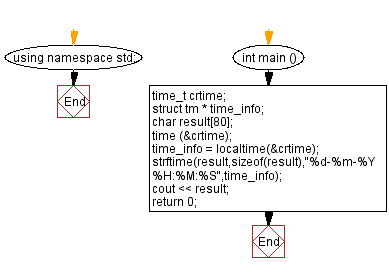
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Date Exercises Home.
Next: Get the day of the week from a given date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/date/cpp-date-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics