C++ Exercises: Display the operation of pre and post increment and decrement
Pre and Post Increment/Decrement
Write a C++ program to display the operation of pre and post increment and decrement.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int num = 57; // Initializing an integer variable 'num' with the value 57
cout << "\n\n Display the operation of pre and post increment and decrement :\n"; // Outputting a message for demonstrating increment and decrement operations
cout << "--------------------------------------------------------------------\n"; // Outputting a separator line
cout <<" The number is : " << num << endl; // Displaying the initial value of 'num'
num++; // Post-incrementing 'num' by 1
cout <<" After post increment by 1 the number is : " << num << endl; // Displaying 'num' after post-incrementing
++num; // Pre-incrementing 'num' by 1
cout <<" After pre increment by 1 the number is : " << num << endl; // Displaying 'num' after pre-incrementing
num = num + 1; // Increasing 'num' by 1
cout <<" After increasing by 1 the number is : " << num << endl; // Displaying 'num' after increasing by 1
num--; // Post-decrementing 'num' by 1
cout <<" After post decrement by 1 the number is : " << num << endl; // Displaying 'num' after post-decrementing
--num; // Pre-decrementing 'num' by 1
cout <<" After pre decrement by 1 the number is : " << num << endl; // Displaying 'num' after pre-decrementing
num = num - 1; // Decreasing 'num' by 1
cout <<" After decreasing by 1 the number is : " << num << endl; // Displaying 'num' after decreasing by 1
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Display the operation of pre and post increment and decrement : -------------------------------------------------------------------- The number is : 57 After post increment by 1 the number is : 58 After pre increment by 1 the number is : 59 After increasing by 1 the number is : 60 After post decrement by 1 the number is : 59 After pre decrement by 1 the number is : 58 After decreasing by 1 the number is : 57
Flowchart:
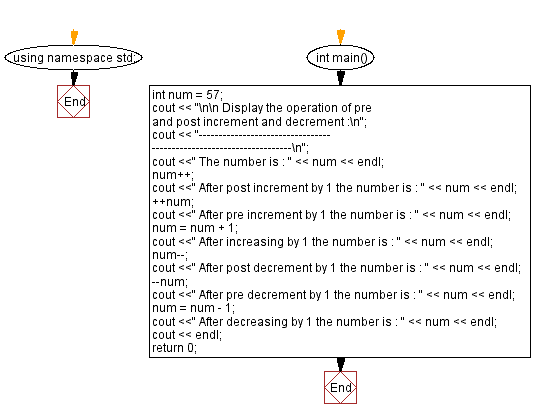
C++ Code Editor:
Previous: Write a program in C++ to check overflow/underflow during various arithmetical operation.
Next: Write a program in C++ to formatting the output.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics