C++ Exercises: Convert a given number into hours and minutes
Convert Number to Hours and Minutes
Write a C++ program to convert a given number into hours and minutes. Separate the number of hours and minutes with a colon.
For example if a given number is 67 the output should be 1:7
Visual Presentation:
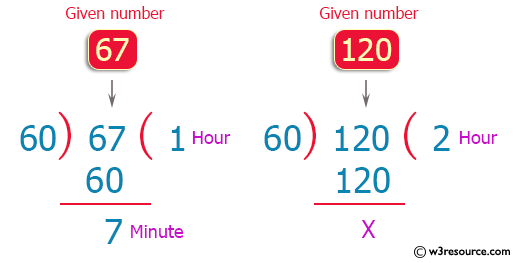
Sample Solution:
C++ Code :
#include <iostream>
#include <string>
using namespace std;
// Function to convert a number to hours and minutes
void Time_Convert(int num) {
bool flag; // Flag to control loop
int hr = 0; // Initialize hours to 0
do {
flag = false; // Set flag to false initially
if (num >= 60) {
hr++; // Increment hours
num -= 60; // Subtract 60 from the number
flag = true; // Set flag to true to continue the loop
}
} while (flag); // Continue loop until flag is false
// Print the converted time in "H:M" format
cout << "\nH:M " << hr << ":" << num << endl;
}
int main() {
// Function calls to convert different numbers to hours and minutes
Time_Convert(67);
Time_Convert(60);
Time_Convert(120);
Time_Convert(40);
return 0;
}
Sample Output:
H:M 1:7 H:M 1:0
Flowchart:
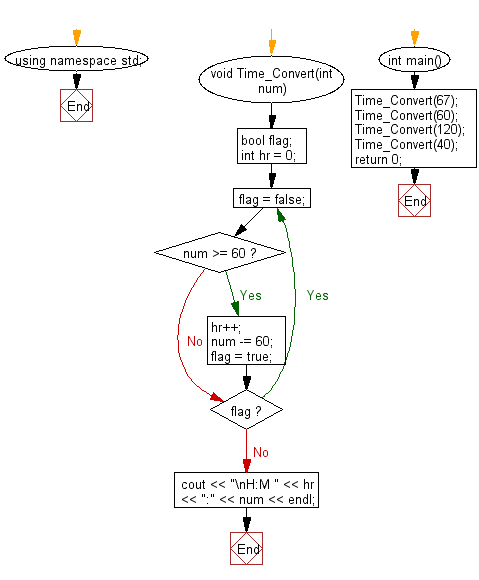
C++ Code Editor:
Previous: Write a C++ program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
Next: Write a C++ program to check whether the sequence of the numbers in a given array is a “Arithmetic” or “Geometric” sequence.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics