C++ Exercises: Prints the word and a list of the corresponding page numbers
C++ Basic: Exercise-82 with Solution
Write a C++ program that reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
Sample Solution:
C++ Code :
#include <iostream>
#include <vector>
#include <algorithm> // Required for sort()
using namespace std;
// Define a pair alias for string and int
typedef pair<string, int> P;
int main()
{
string str;
int page, ctr = 0;
vector<P> v_data; // Vector of pairs to store string and int pairs
// Input loop: Read string and integer pairs until the end of input
while (cin >> str >> page) {
v_data.push_back(P(str, page)); // Store the pair (string, int) in the vector
ctr++;
}
// Sort the vector of pairs based on string (lexicographical order)
sort(v_data.begin(), v_data.end());
// Loop through the sorted vector of pairs
for (int i = 0; i < ctr; i++) {
if (i == 0) {
cout << v_data[i].first << endl; // Print the first string
cout << v_data[i].second; // Print the first associated integer
continue;
}
// Check if the current string is the same as the previous string
if (v_data[i].first == v_data[i - 1].first && v_data[i].second != v_data[i - 1].second) {
cout << ' ' << v_data[i].second; // If the strings match but integers don't, print the current integer
continue;
} else {
cout << endl; // Print a newline when the strings differ
}
cout << v_data[i].first << endl; // Print the current string
cout << v_data[i].second; // Print the associated integer
}
puts(""); // Print a newline at the end
return 0;
}
Sample Output:
Sample Input: Python 2 HTML 4 CSS 3 Python 5 Python 3 HTML 2 CSS 6 Output: CSS 3 6 HTML 2 4 Python 2 3 5
Flowchart:
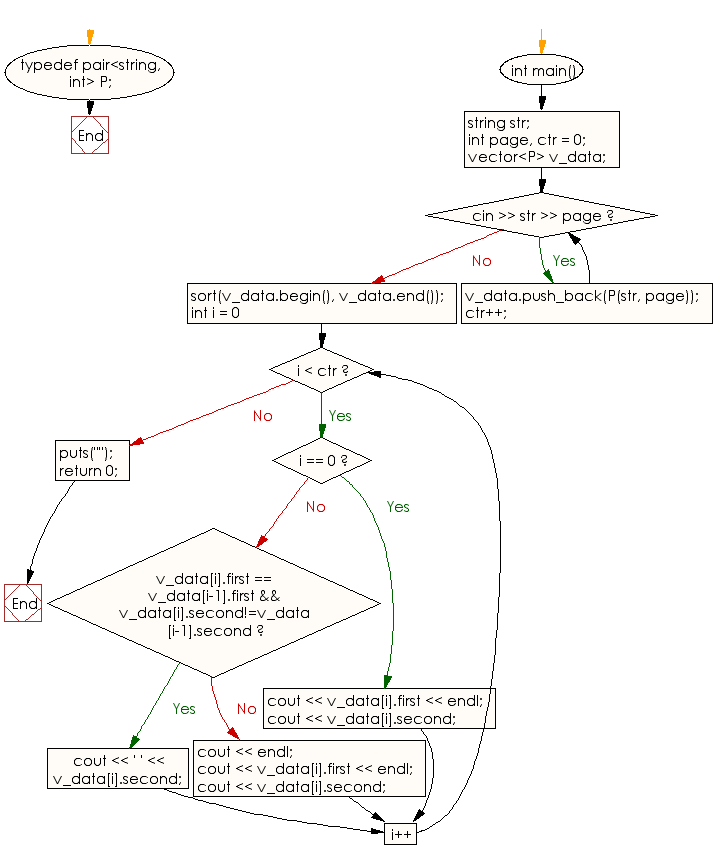
C++ Code Editor:
Previous: Write a C++ program to which replace all the words "dog" with "cat".
Next: Write a C++ program to convert a given number into hours and minutes. Separate the number of hours and minutes with a colon.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic/cpp-basic-exercise-82.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics